Golang, known for its simplicity and high performance, is increasingly becoming the preferred choice for developing scalable applications. Mastering Golang interview questions is crucial for developers aiming to take the next step in their career. Many Go interview questions test your understanding of Go’s unique features, including concurrency, error handling, and memory management. They are designed to assess your coding skills and ability to solve real-world problems effectively.
At Aloa, our project management system and network of vetted professionals ensure you’re equipped to handle complex Go challenges. From structuring projects to debugging and optimization, we provide the support you need to grow your expertise and perform at your best.
To help you prepare, we’ve compiled 13 must-know Golang interview questions covering slices, error handling, concurrency, and more.
Let’s dive in!
Go Language Syntax and Features
The Go programming language simplifies software development with clean syntax, efficient concurrency through goroutines and channels, and a robust standard library. Renowned for scalable and high-performance applications, Go code is a focal point in Golang interview questions, especially for developers building APIs, distributed systems, and microservices.
A Go source file contains the code necessary to build applications, organized within a Go workspace and managed by Go modules. These modules, defined by go.mod, ensure dependency, and consistency, simplifying package management. Key environment variables like the Goroot variable specify the Go compiler and core libraries, which form the foundation for building reliable applications.
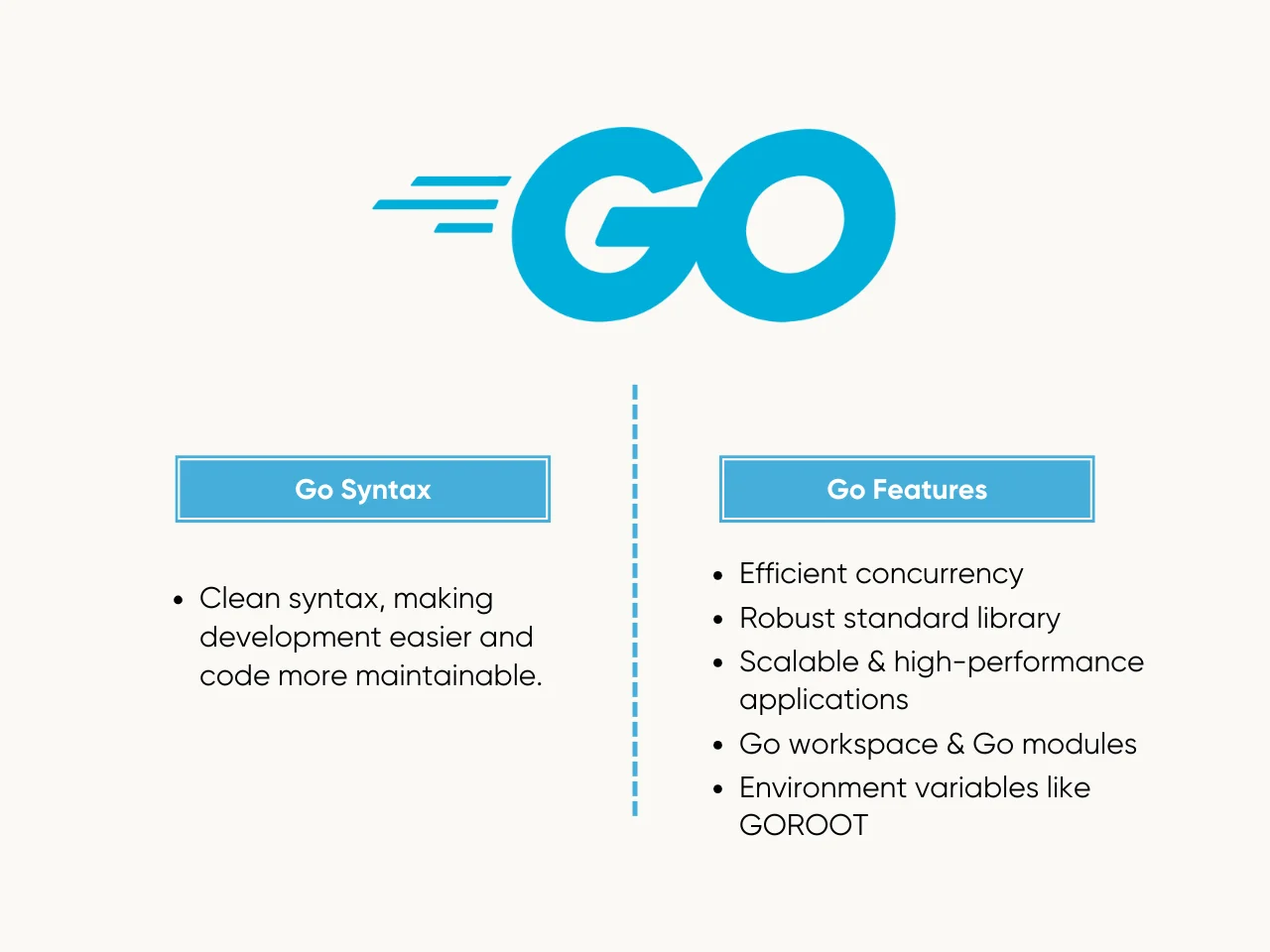
The main() function is the entry point for executing Go programs. This structure keeps projects well-organized, making it easier for developers to manage linked packages and maintain a clean, modular codebase.
What are slices in Go, and how do they differ from arrays?
Slices in Go are dynamic, resizable data structures that are more flexible than arrays, which have a fixed size. Unlike arrays, slices reference an underlying array which can enable multiple slices to share the same memory and reduce redundancy.
To better understand the flexibility and capabilities of slices, let’s break down their key differences from arrays:
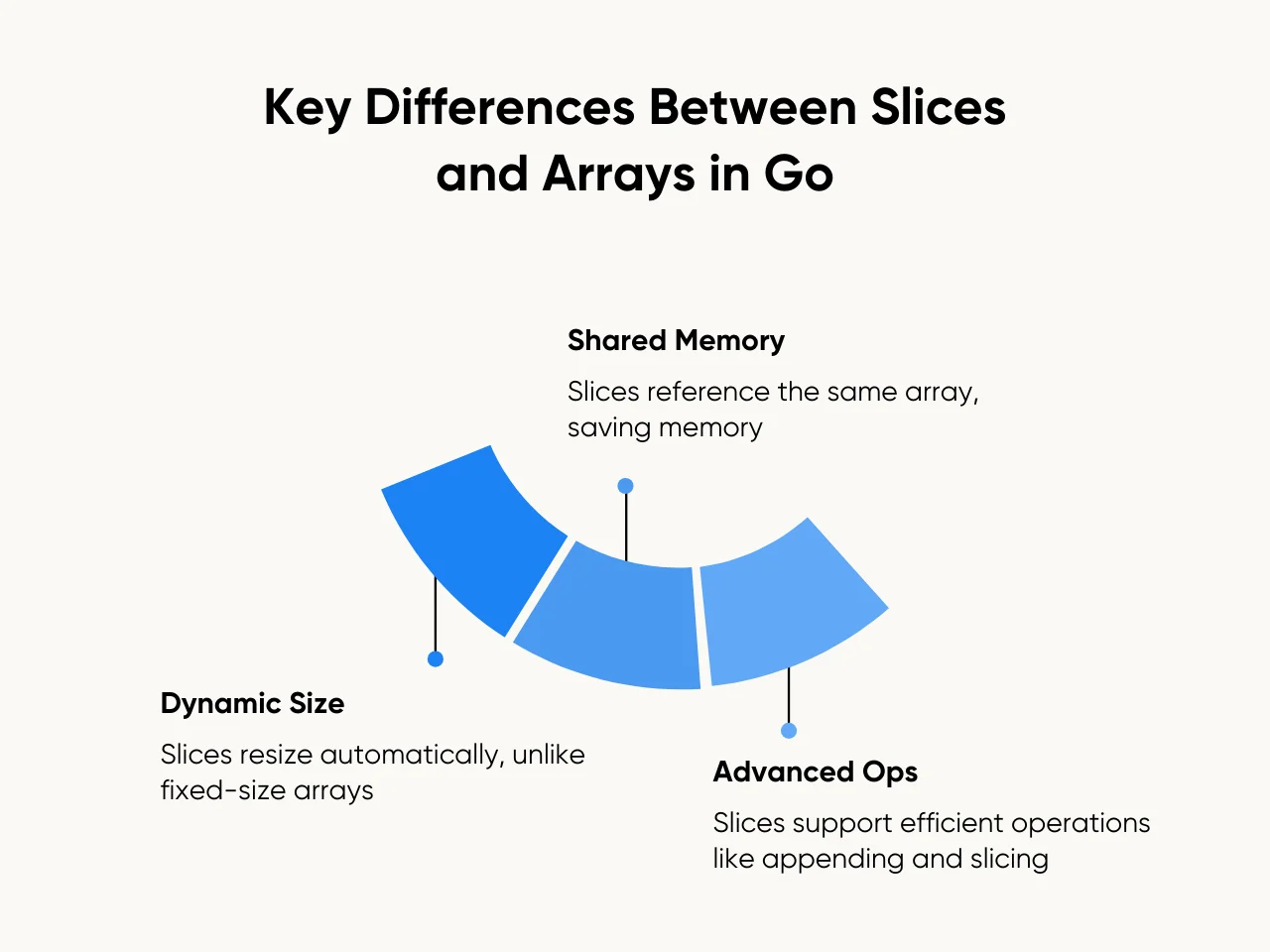
- Dynamic Size and Flexibility: Slices can dynamically resize to accommodate additional elements. Arrays are fixed during declaration.
- Shared Memory: Multiple slices can reference the same array, reducing redundancy and enhancing performance during data manipulation. Arrays, on the other hand, do not share memory by default.
- Support for Advanced Operations: Slices support appending, copying, and slicing subsets of data, simplifying data processing tasks. Arrays require manual implementation for these operations.
How does the Go garbage collector work?
Go’s Garbage Collector (GC) plays a critical role in automated memory management for performance-oriented applications: it automates memory management by reclaiming unused resources, preventing leaks, and ensuring efficient utilization.
- Automatic Memory Reclamation: The GC identifies and reclaims unused memory resources, ensuring efficient memory utilization and preventing memory leaks without developer intervention.
- Optimized for Low-Latency Operations: Designed for real-time and high-performance systems, Go’s GC minimizes application pauses during collection, maintaining consistent execution speed.
- Pointer and Reference Handling: The GC effectively manages pointers and references, simplifying complex memory allocation tasks and reducing the likelihood of errors in concurrent applications.
Automatic garbage collection in Golang is notably more efficient than Java or Python, as it executes concurrently alongside the program, improving runtime efficiency and reducing bugs. Golang's ability to speed up code development has made it a popular choice for companies like Google, Apple, and Uber, which is why it’s a key topic in Golang interview questions.
How does Go’s error handling differ from exceptions in other languages?
Go handles errors differently by focusing on explicit error management instead of exceptions. Functions return an error value as their last parameter, requiring developers to handle errors directly and making the control flow more predictable. This approach reduces the risk of overlooking errors and ensures clear communication of success or failure in every function.
As a developer, you should present a solid understanding of Go’s error handling approach during the golang interview.
Here’s an example of error handling with if err != nil and custom errors:
package main
import (
"errors"
"fmt"
)
func divide(a, b int) (int, error) {
if b == 0 {
return 0, errors.New("division by zero is not allowed")
}
return a / b, nil
}
func main() {
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
Concurrency in Golang
Concurrency in Golang is one of Go’s standout features, as it enables efficient and scalable execution of tasks. Go simplifies concurrency with tools like the select statement which helps goroutines handle multiple communication channels at once. Developers can create flexible and reusable code that works well in concurrent programs using interfaces and their method signatures.
What are goroutines, and how are they different from threads?
Goroutines are lightweight functions that are managed by the Go runtime rather than the operating system. They are a fundamental part of Go’s concurrency model.
Lightweight threads, also known as Goroutines in Go, are a key feature that enables concurrency in the programming language.
The differences between goroutines and threads are as follows:
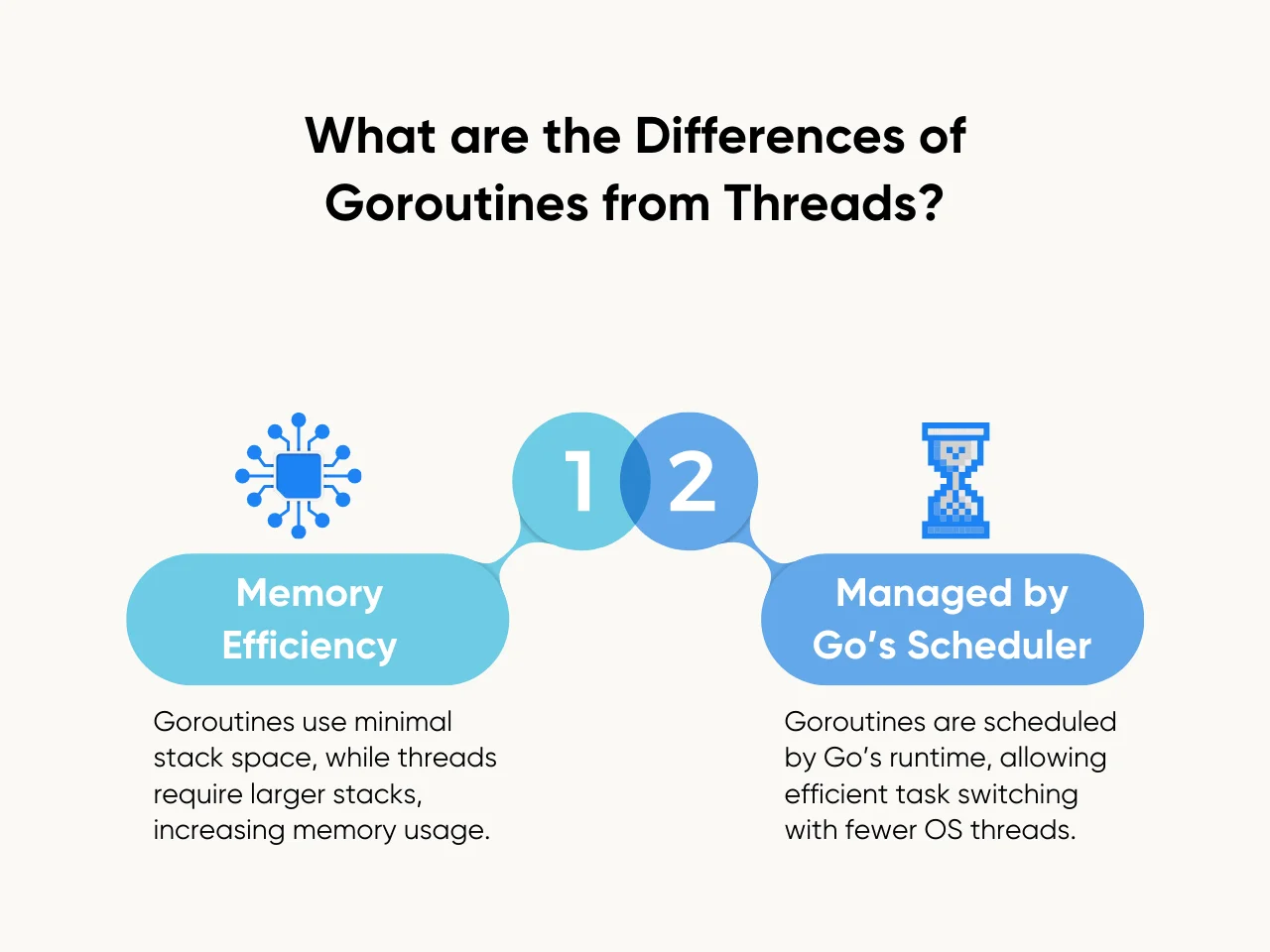
- Memory Efficiency: Goroutines are more memory-efficient than threads, requiring only a few kilobytes of stack space which grows dynamically as needed. Threads, on the other hand, start with a significantly larger stack size, leading to higher memory consumption.
- Managed by Go’s Scheduler: Unlike threads that rely on the operating system for scheduling, goroutines are managed by Go’s runtime scheduler. This enables efficient task switching and allows thousands of goroutines to run on a limited number of OS threads which minimizes context-switching overhead.
Goroutines are initiated using the go keyword. For instance, in a web server, each incoming request can be processed by a separate goroutine:
package main
import (
"fmt"
"time"
)
func printMessage(msg string) {
for i := 0; i < 3; i++ {
fmt.Println(msg)
time.Sleep(500 * time.Millisecond)
}
}
func main() {
go printMessage("Goroutine") // Runs in a goroutine
printMessage("Main Function") // Runs in the main thread
}
The go keyword launches printMessage as a goroutine, allowing it to execute concurrently with the main function. This shows Go’s lightweight concurrency.
How do you avoid race conditions in Go programs?
Race conditions happen when multiple goroutines access and modify shared data at the same time without proper synchronization which causes unpredictable behavior. To avoid race conditions in Go programs, consider these solutions:
- Use sync Primitives (sync.Mutex): A sync.Mutex ensures that only one goroutine can access a critical section of code at a time, preventing data inconsistencies.
- Employ Channels for Safe Communication: Channels allow goroutines to pass data safely without sharing memory directly. This aligns with Go’s philosophy of “communicating by sharing” instead of “sharing memory.”
For instance, in a scenario where multiple goroutines increment a shared counter, a sync. Mutex can be used to lock and unlock the critical section, ensuring that only one goroutine modifies the counter at a time. Alternatively, channels can be employed to safely pass data between goroutines which removes the need for shared memory and eliminates the risk of race conditions.
What are Go channels, and how do they facilitate communication?
Go channels are strongly typed conduits used for communication between goroutines. They allow data of a specific type to be passed safely and efficiently, ensuring type safety and synchronization. Channels are a key feature of Go's concurrency model and are frequently discussed in Golang interview questions for developers focused on building synchronized and efficient systems.
Channels in Go can operate in two modes:
- Unbuffered channels synchronize the sender and receiver, requiring both to be ready at the same time for the data transfer.
- Buffered channels allow data to be sent and stored temporarily without requiring an immediate receiver, enabling asynchronous communication.
Goroutines can exchange data without accessing shared memory directly by using channels. This eliminates the need for locking mechanisms like sync.Mutex which reduces the risk of race conditions and deadlocks.
An example of sending and receiving data between goroutines can demonstrate how channels facilitate communication:
package main
import "fmt"
func main() {
// Creating an unbuffered channel
ch := make(chan string)
// Goroutine to send data into the channel
go func() {
ch <- "Hello, Channel!"
}()
// Receiving data from the channel
message := <-ch
fmt.Println(message) // Output: Hello, Channel!
}
Go’s Standard Library and Tooling
The Go standard library provides developers with the tools to build efficient and scalable applications while minimizing the need for external dependencies. It simplifies tasks such as building web servers, managing concurrent operations, and organizing projects. Mastering the Go standard library is essential for solving real-world development challenges and is a common focus in Golang interview questions.
The library excels in handling data types, such as string literals, raw string literals, and numeric values, making it easy to work with structured and unstructured data. It also supports advanced features like type conversion, type assertion, and type switch which enables developers to manage complex logic with minimal effort.
Tools for working with struct values enhance data organization within applications, while the compilation process efficiently converts Go code into machine code, ensuring optimal performance at the time of compilation.
These features make the standard library indispensable for writing clean, maintainable, and high-performance Go programs.
How does the net/http package simplify building web servers?
The net/http package simplifies web server development by providing a fully functional HTTP server with minimal setup. Developers can start a server in just a few lines of code without relying on external dependencies. It allows custom routing through handlers, with functions like http.HandleFunc
making it easy to link URLs to specific actions.
The package also supports extensibility and middleware integration, enabling features like logging, authentication, and data validation:
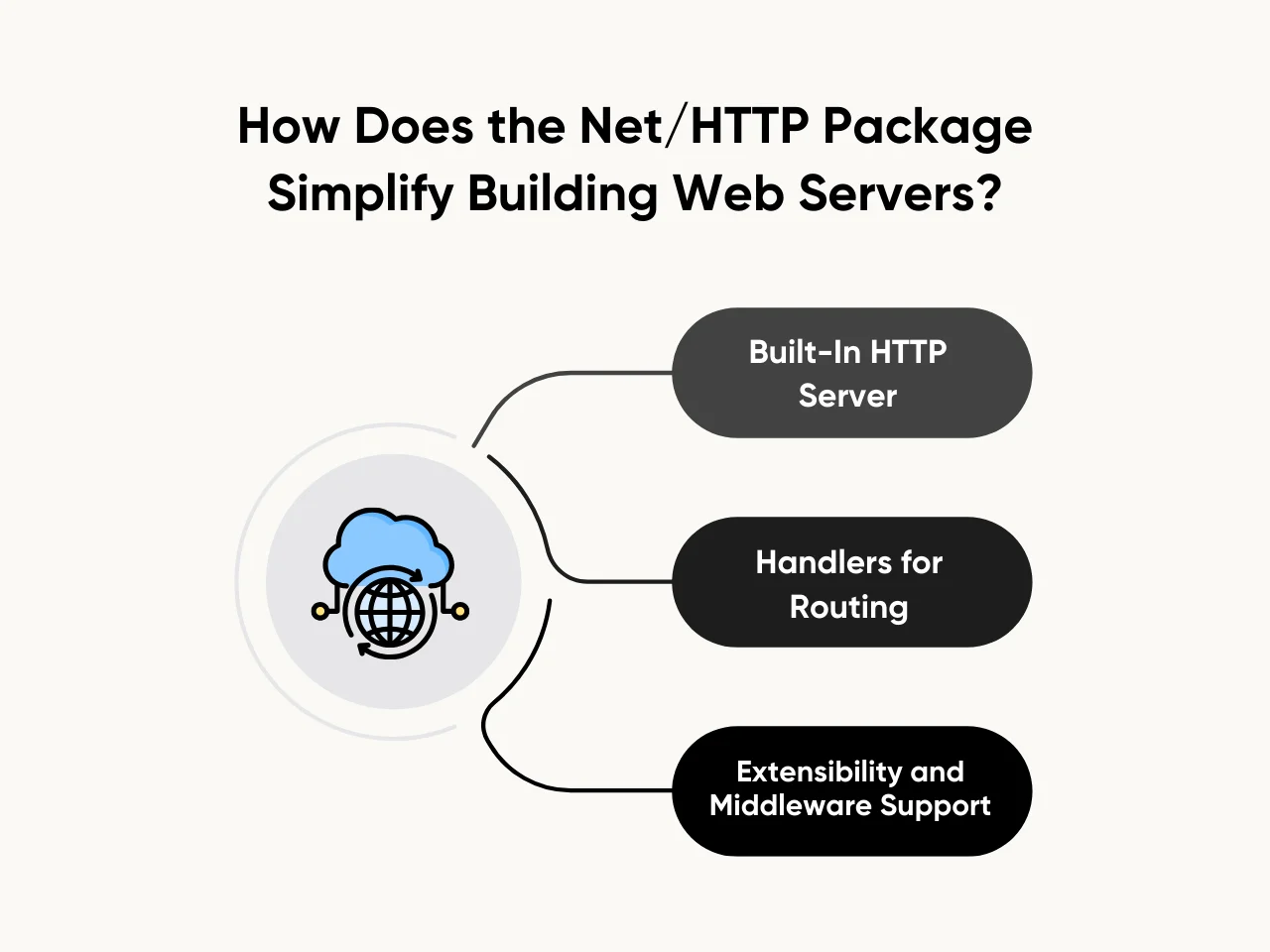
- Built-In HTTP Server: The package provides a fully functional HTTP server that can be started with just a few lines of code, eliminating the need for additional setup or dependencies.
- Handlers for Routing: Developers can define custom handlers for routing requests and processing responses. Functions like http.HandleFunc simplify associating URLs with specific actions, streamlining the development of RESTful APIs.
- Extensibility and Middleware Support: The net/http package integrates seamlessly with middleware, enabling additional functionalities like logging, authentication, and data validation.
For example, to create a simple HTTP server that handles GET requests, you can use the following:
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, you've reached %s!", r.URL.Path)
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
What is the purpose of the context package?
The context package in Go is designed to address key needs in concurrent programming, particularly for managing deadlines, cancellations, and passing request-scoped data. It is especially useful in networked applications and distributed systems:
- Managing Deadlines and Timeouts: The package allows developers to specify deadlines for operations, ensuring that long-running tasks do not consume resources indefinitely.
- Cancellation Propagation: When a parent operation is canceled, the context package ensures that all child processes or requests are also terminated, freeing up resources and preventing cascading failures.
- Request-Scoped Data: Context enables the safe passage of data, like authentication tokens or user information, across different layers of an application during a request’s lifecycle.
Example of Using context.WithTimeout
to Enforce Timeouts:
package main
import (
"context"
"fmt"
"time"
)
func main() {
ctx, cancel := context.WithTimeout(context.Background(), 2*time.Second)
defer cancel()
select {
case <-time.After(3 * time.Second):
fmt.Println("Operation completed")
case <-ctx.Done():
fmt.Println("Timeout exceeded:", ctx.Err())
}
}
How do you structure and organize Go projects?
Adopting standard layouts and clear naming conventions helps teams work efficiently and avoid technical debt:
- Follow Standard Project Layouts: Use common directories like
pkg/
for reusable code,cmd/
for application entry points, andinternal/
for non-exported logic. - Meaningful Package Names: Ensure package names are intuitive and descriptive, reflecting their purpose without redundancy.
- Separation of Concerns: Divide functionality across dedicated folders such as
handlers/
for request handlers,models/
for data structures, andservices/
for business logic. This modular approach enhances readability and simplifies testing.
For example, organizing a REST API project:
cmd/
: Contains the main entry point for the application.pkg/
: Houses reusable libraries or utility functions shared across the project.internal/
: Contains non-exported code specific to the application.handlers/
: Defines HTTP handlers that process incoming requests and responses.models/
: Represents data structures, such as database entities or API response schemas.services/
: Implements the core business logic, connecting handlers to data sources.
Go developers can maintain clarity in their codebase by adhering to this structure thus enabling easier debugging, testing, and scaling as the project grows. This is particularly valuable in team environments where clear organization reduces onboarding time and misunderstandings.
Debugging, Testing, and Optimization
Go provides extensive support for debugging, testing, and performance optimization, making it easier for developers to write robust and efficient code. Features like these are naturally posed in Golang interview questions because they showcase how Go excels in handling real-world development challenges.
How do you write tests in Go?
Go uses its built-in testing package to write and run tests. Developers can write unit tests to verify individual functions and ensure they work as expected. For better coverage and efficiency, table-driven tests are used to test multiple scenarios in a single function by iterating over structured data.Go offers several approaches for writing effective tests, including the following:
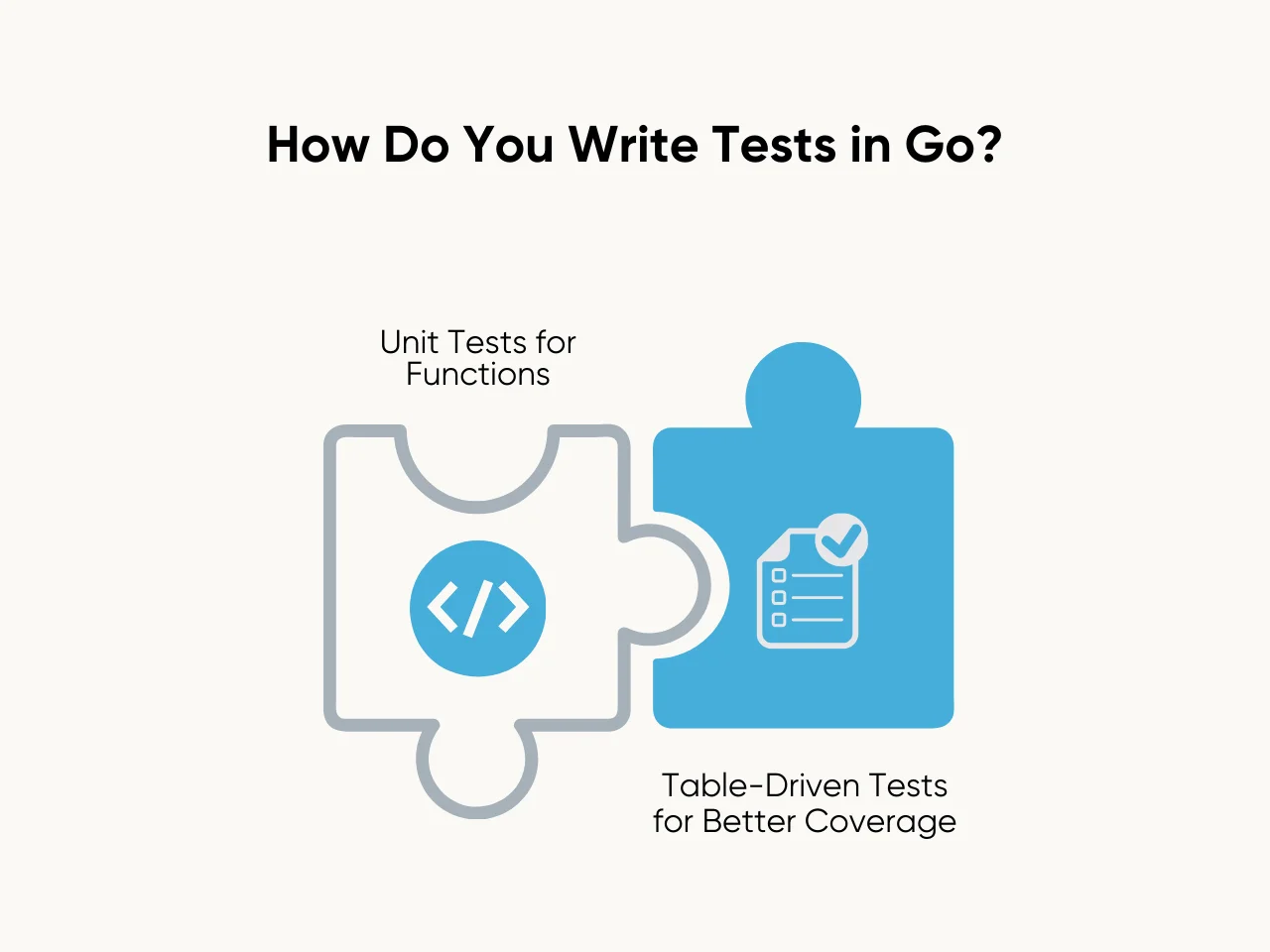
- Unit Tests for Functions: These tests focus on individual functions to ensure they behave as expected. They form the foundation of test coverage.
- Table-Driven Tests for Better Coverage: Table-driven tests use structured data to test multiple cases within a single function, improving test efficiency and readability.
Example: Writing a Test with t.Run()
The t.Run
function allows subtests to be grouped under a parent test, simplifying complex testing scenarios.
package main
import (
"testing"
)
func Sum(a, b int) int {
return a + b
}
func TestSum(t *testing.T) {
testCases := []struct {
name string
inputA int
inputB int
expected int
}{
{"both positive", 2, 3, 5},
{"positive and negative", 5, -3, 2},
{"both zero", 0, 0, 0},
}
for _, tc := range testCases {
t.Run(tc.name, func(t *testing.T) {
result := Sum(tc.inputA, tc.inputB)
if result != tc.expected {
t.Errorf("expected %d, got %d", tc.expected, result)
}
})
}
}
This test function validates the behavior of the Sum function for multiple scenarios using table-driven tests.
What tools do you use to profile and optimize Go applications?
Optimizing Go applications involves identifying performance bottlenecks and ensuring concurrent safety. Go provides built-in tools like pprof
and the race detector to address these challenges:
pprof
for CPU/Memory Profiling: The pprof tool analyzes an application’s CPU and memory usage, helping developers identify slow or resource-intensive code paths. Profiling generates detailed reports that can pinpoint inefficiencies in function execution or memory allocation.- Go Race Detector for Identifying Race Conditions: Race conditions occur when multiple goroutines access shared resources simultaneously without proper synchronization. The race detector (
go test -race
) scans concurrent programs to detect potential issues, ensuring safe data access.
To detect race conditions, run the following command:
go test -race ./...
This command runs all tests in the current directory with the race detector enabled. It reports any conflicts in accessing shared resources and helps developers fix concurrency issues before deployment.
How do you debug Go applications?
Debugging Go applications requires the right tools and techniques to identify and resolve issues efficiently. Developers can leverage interactive debugging tools, logging mechanisms, and integrated development environments to effectively inspect code behavior and address errors.
To debug a Go application using Delve, you start the debugger and set breakpoints at the parts of the code where you think the problem might be. For instance, you might set a breakpoint at the initialization of a pointer variable or during the modification of a global variable to examine how its memory address or value changes. When the program runs and reaches a breakpoint, it pauses, allowing you to check the values of variables and see how the program is behaving at that point.
You can then move through the code step by step to find where things go wrong and fix the issue. This method helps you clearly understand what your program is doing and why it might not be working as expected, especially when managing variable declarations, inspecting special variables, or verifying memory usage.
Tools for debugging Go applications include:
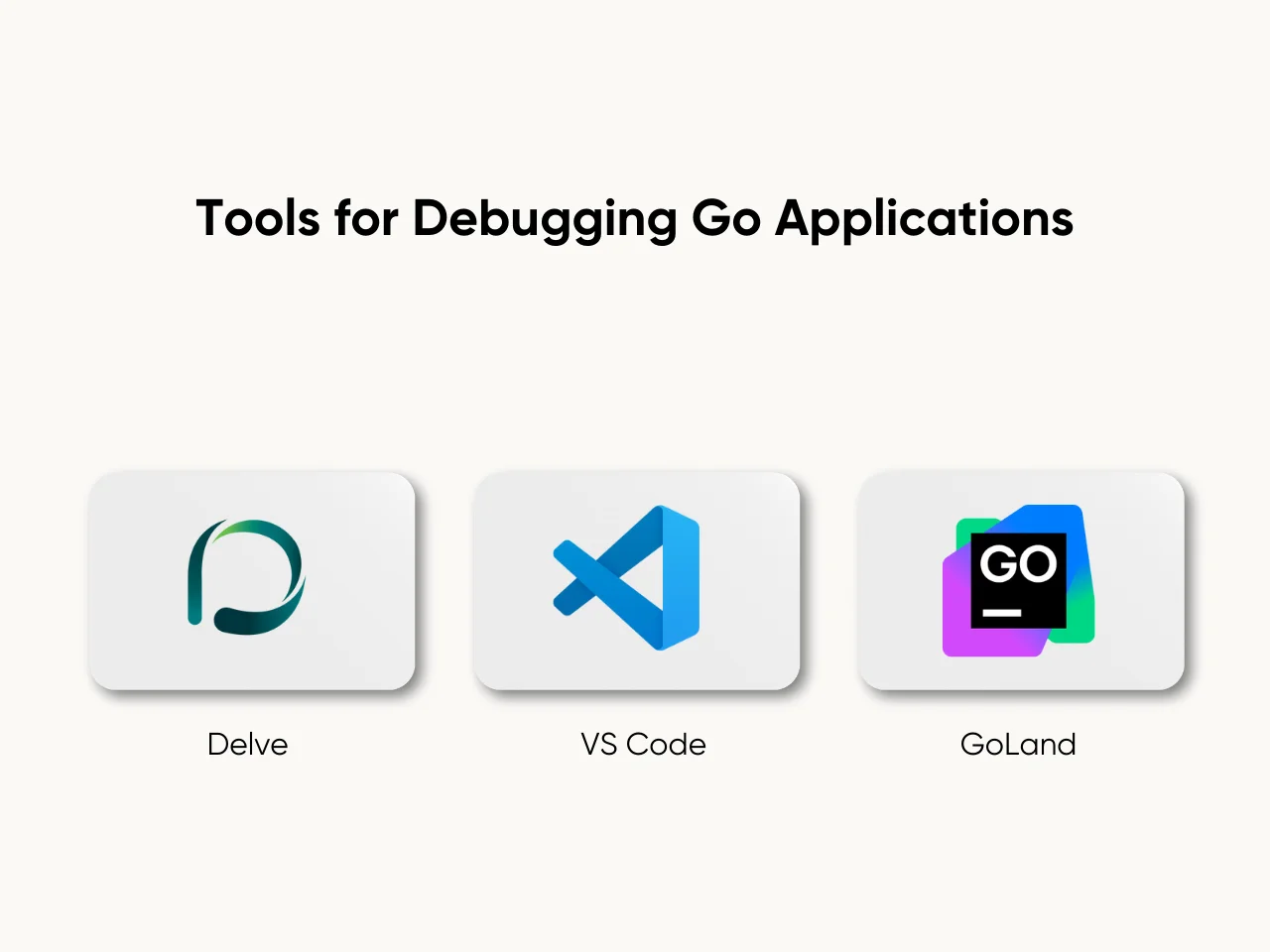
- Delve (
dlv
): Delve is a powerful debugger designed specifically for Go programs. It allows developers to inspect variables, set breakpoints, and step through code line-by-line to identify issues during runtime. For example, you can use Delve to analyze global variables, examine the memory address or pointer variable of a specific structure, and monitor how the program changes the values of these variables during execution. - Integrated Debugging in IDEs: Popular Integrated Development Environments (IDEs) like VS Code and GoLand provide built-in debugging support for Go. These IDEs seamlessly integrate with Delve, offering a user-friendly interface for inspecting code behavior and tracking errors. They also simplify debugging processes, such as monitoring the declaration and usage of special variables or understanding how the memory address of variables is utilized in a running program.
Developers can seamlessly debug applications utilizing Delve and IDE integrations thus ensuring their programs are reliable and perform as intended.
Preparing for Intermediate-Level Interviews
When preparing for intermediate-level Go interviews, showcasing your expertise and demonstrating a strong grasp of Go's design principles can significantly impact your success. Mastering how to highlight your skills and articulate trade-offs in Go’s design is essential for standing out as a candidate.
How do you highlight your Go experience in an interview?
Highlighting your Go experience in interviews involves emphasizing the skills and projects that demonstrate your ability to solve real-world challenges:
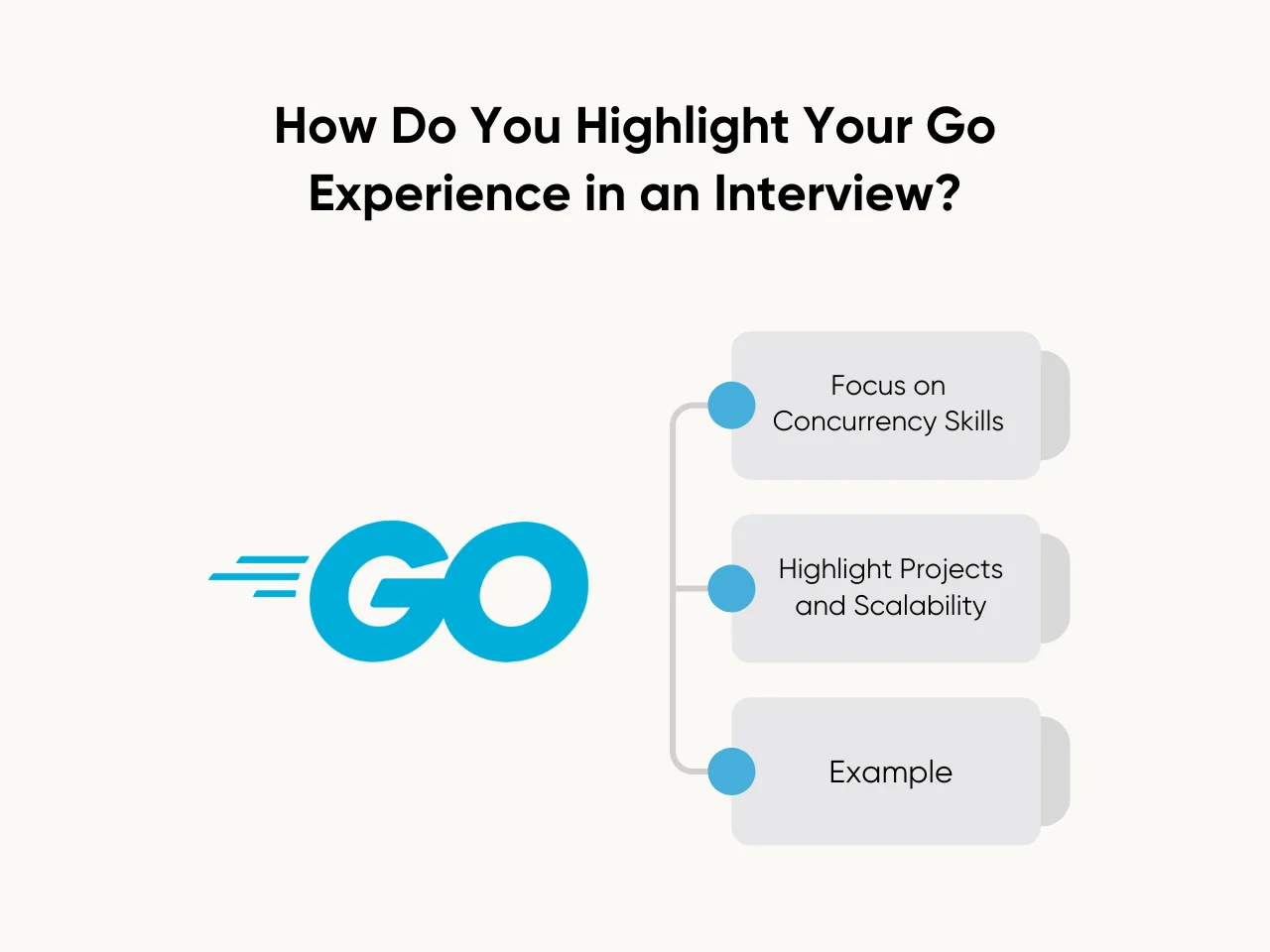
- Focus on Concurrency Skills: Emphasize your expertise with Go's concurrency model, including goroutines and channels. Highlighting your ability to manage parallel tasks using Go's unique features efficiently is key, as concurrency is a critical area in Golang interview questions.
- Highlight Real-World Projects and Scalability Achievements: Share specific examples where you applied Go to address complex challenges, focusing on measurable results. For instance, discuss optimizing an API for higher performance or scaling a service to handle increased traffic.
- Example: "I optimized our API’s concurrency model by leveraging goroutines and channels, reducing response times by 40% and enhancing scalability to handle peak traffic efficiently."
How do you explain trade-offs in Go’s design?
Explaining trade-offs in Go’s design demonstrates your understanding of the language's principles and how they align with development goals. Interviews often explore this to assess your ability to make informed decisions when designing applications:
- Simplicity and Performance vs. Missing Features:
- Go emphasizes simplicity and runtime efficiency, sometimes at the expense of advanced features. For example, Go avoided generics until version 1.18 to maintain straightforward syntax and minimal complexity.
- Explicit Error Handling vs. Exception-Based Systems:
- Go's error handling approach, which relies on explicit return values, promotes clarity and control over the program flow. However, this contrasts with exception-based systems that may be more concise but often hide errors.
- Example: "Go’s explicit error handling enhances maintainability in large projects by making error states visible and predictable. While it may introduce verbosity, it reduces hidden bugs and supports a more robust codebase."
Key Takeaway
Understanding the Go concepts not only showcases your skills but also gives you the tools to handle challenges in Go development. That’s why such concepts are also included in Golang interview questions as they test your ability to work effectively with Go’s key features, such as concurrency, error handling, and efficient memory management. Are your concurrency solutions optimized to avoid race conditions? Is your approach to project organization scalable and maintainable? These are the types of questions that separate basic knowledge from true expertise in Go.Aloa is here to help you strengthen your Go skills and navigate these challenges with ease. With a network of vetted professionals and expert-backed tools, we provide the guidance you need to grow and succeed. Visit Aloa Blog today to advance your Go development journey.