C++ remains a cornerstone in the programming language landscape, particularly for software development, systems programming, and game development. Its flexibility and efficiency make it a preferred extension of the C programming language, enabling developers to manage memory space effectively and optimize performance. Experienced developers must master concepts like runtime polymorphism, operator overloading, and memory management to thrive in C++ interview questions.
At Aloa, we ensure software developers access high-quality resources for their projects. We utilize a robust project management framework with transparent reporting and industry expertise. Our software development teams excel at handling large objects, optimizing source code, and delivering results using the latest trends. Aloa’s commitment lies in guiding you through every step of the software development process.
Building on proven strategies, we’ve created this guide to help experienced developers master critical C++ interview questions. We'll explore the core C++ concepts for experienced developers, advanced object-oriented programming (OOP) concepts, memory management and performance, templates and generic programming, and concurrency and multithreading. By the end, you’ll gain confidence in tackling complex C++ technical interview questions and solidify your expertise in this foundational programming language.
Let's get started!
Core Concepts C++ Interview Questions
Understanding core concepts is essential when tackling C++ interview questions for experienced developers. In interviews, topics like memory management, data structures, and the differences between pointers and references often appear. These questions test fundamental knowledge crucial for solving real-world problems.
Here are the three key concepts every developer should master:
1. What are the differences between pointers and references in C++?
Pointers and references differ in their functionality and usage in C++. Pointers store the address of a variable and allow manipulation through dereferencing. They can point to nullptr and support arithmetic operations. On the other hand, references act as aliases for existing variables and cannot be null or reassigned. Unlike pointers, references simplify syntax and enforce better safety.
Here's the comparison:
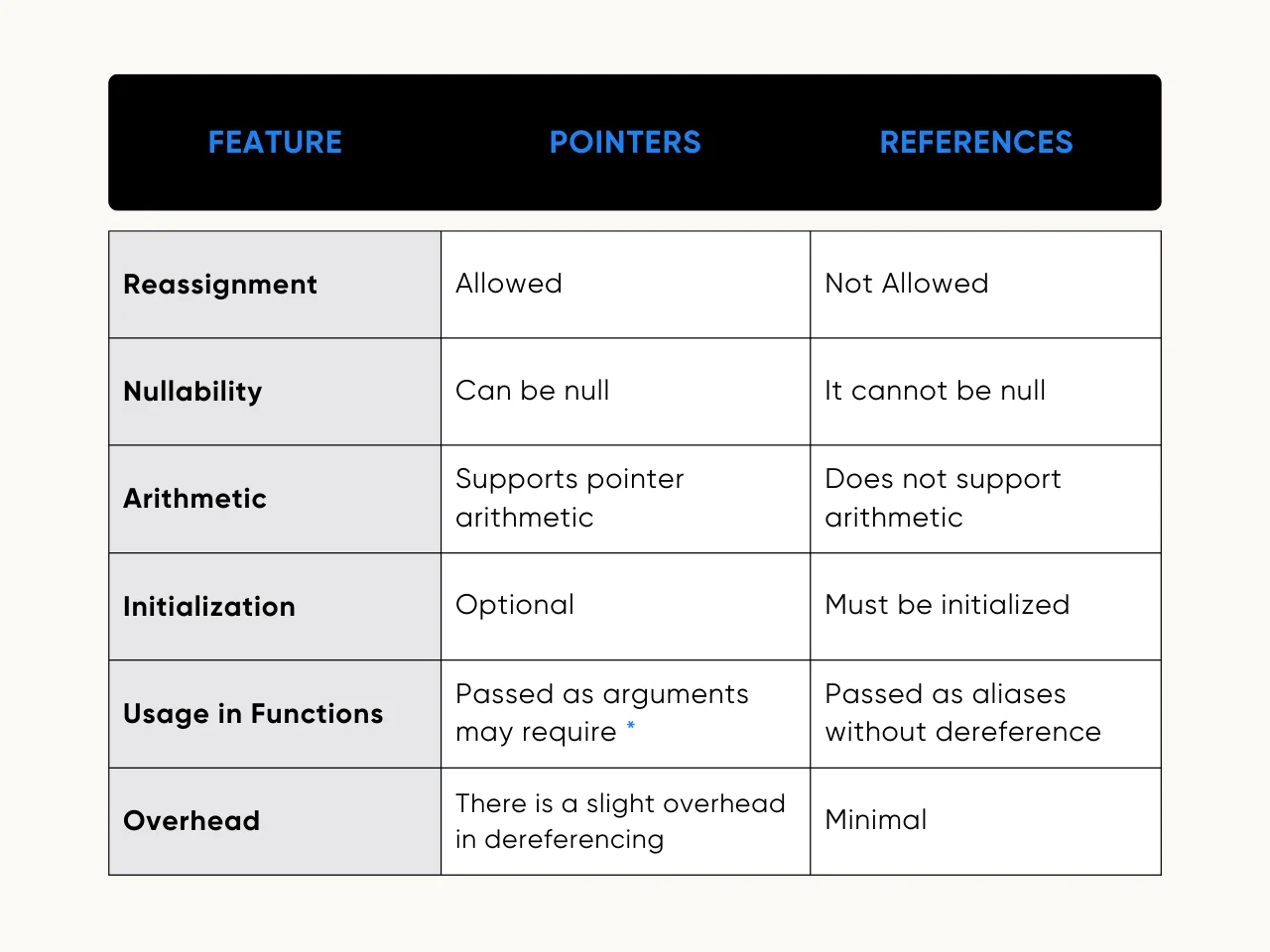
When managing resources dynamically, use a pointer, such as creating a new object int x and manually freeing memory in a c program. References are ideal for passing arguments to member functions, especially in function overloading scenarios or when working with a pure virtual function in a child class.
For instance, a default copy constructor in class d might use references for efficiency, ensuring resource safety when dealing with scope resolution operator or static member function implementations.
2. Explain the rule of three, five, and zero in C++.
The rule of three, five, and zero plays a crucial role in resource management in C++. Developers must understand these rules to write efficient and bug-free code. The rule of three ensures proper handling of resource ownership using the destructor, copy constructor, and copy assignment operator, especially when managing dynamic memory. It also prevents issues during run time when dealing with pointers or reference variables.
Here are the rules explained:
- Rule of Three: A class managing resources must implement a destructor, copy constructor, and copy assignment operator. These ensure proper handling when objects are copied or destroyed.
- Rule of Five: Add a move constructor and move assignment operator for modern C++ (C++11 and later). This enables efficient resource transfer, optimizing performance during compile time.
- Rule of Zero: Classes should rely on RAII principles, such as using smart pointers and avoiding manual memory management. This reduces complexity and enhances reliability.
Developers should also handle friend functions, virtual destructors, and access modifiers effectively. Proper use of storage class, namespace std, and constructors ensures smooth virtual calls. These practices align with any job description requiring expertise in C++ development, allowing for efficient handling of class names and resource management strategies.
3. What is RAII, and why is it important in C++?
RAII, or Resource Acquisition Is Initialization, is a design pattern in C++ that ties a resource's lifecycle to an object's lifetime. This pattern ensures proper resource allocation and deallocation, simplifies memory management, and avoids leaks. Here are the key applications of RAII:
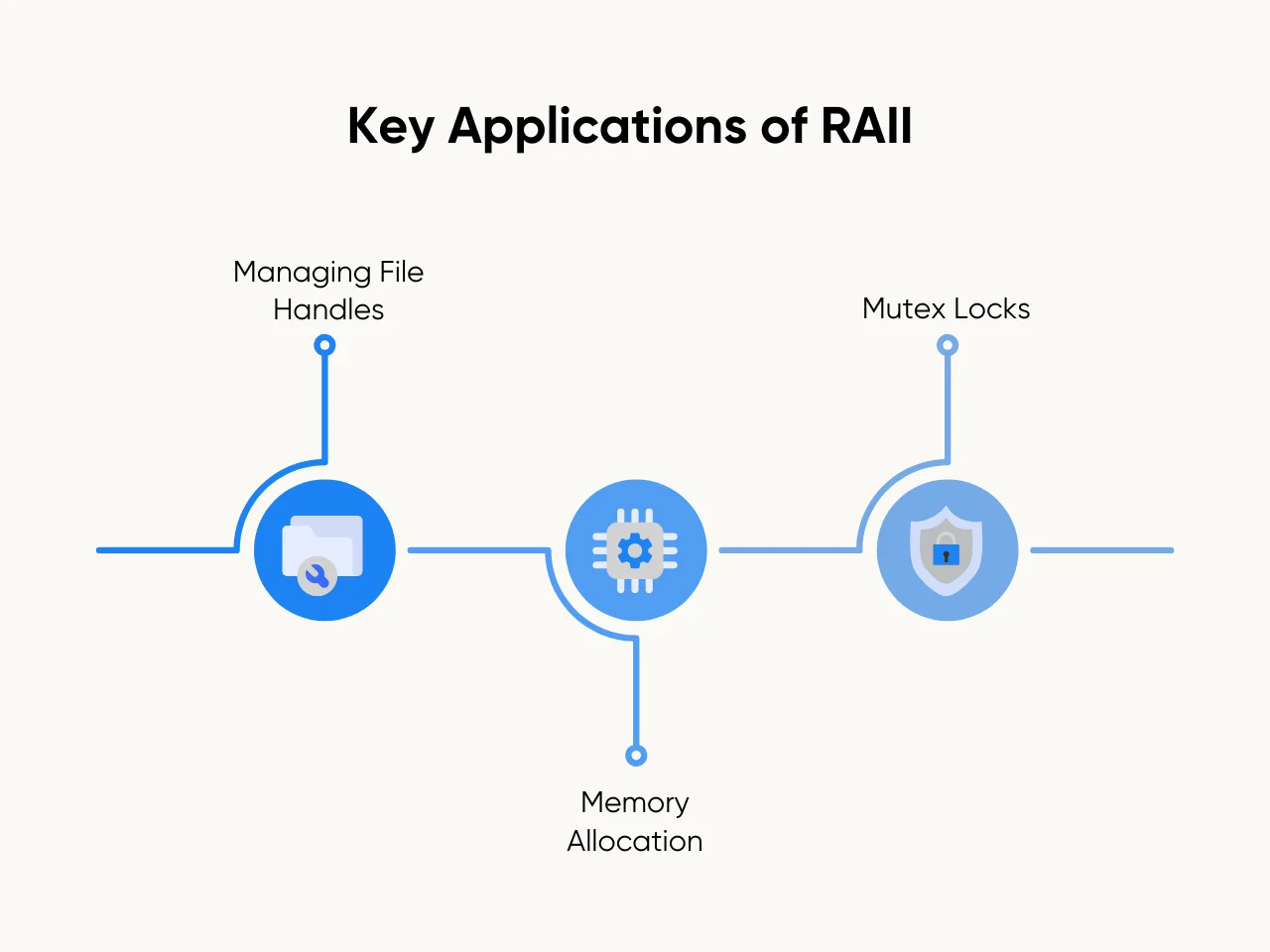
- Managing File Handles: This function automatically closes files when objects go out of scope, ensuring no resource remains open.
- Memory Allocation: Allocates and deallocates memory seamlessly, reducing manual intervention and the risk of memory leaks.
- Mutex Locks: Safely acquires and releases locks in multithreaded applications to prevent deadlocks or resource contention.
RAII is essential for robust C++ programs. It encourages clean, efficient code by ensuring resources are automatically freed when objects are destroyed. Adopting RAII minimizes errors caused by manual resource management and improves program stability. This pattern frequently features in C++ interview questions, showcasing a developer's understanding of best practices in resource handling.
Advanced Object-Oriented Programming (OOP) Concepts
Mastering advanced OOP concepts is critical for answering C++ interview questions effectively. These topics, including runtime polymorphism, virtual functions, and abstract classes, reveal expertise in designing scalable, reusable code. Proficiency in these areas ensures solid problem-solving abilities.
Here are the three must-know OOP concepts for developers:
4. How does polymorphism work in C++?
Polymorphism in C++ allows objects to take on multiple forms, enabling flexibility and scalability in code. This concept ensures a single interface works for underlying data types or classes. Polymorphism improves maintainability, making it a critical topic in C++ interview questions.
These are the two types of polymorphism in C++:
- Static Polymorphism: Achieved through function overloading and templates. Function overloading allows defining multiple functions with the same name but different parameter types. Templates enable the creation of functions or classes that work with any data type, promoting reusability.
- Dynamic Polymorphism: Implemented using virtual functions and inheritance. Virtual functions allow derived classes to override methods in a base class, ensuring the correct function executes during runtime. Inheritance enables reusing and extending existing class functionality.
Abstract base classes with virtual methods ensure extensibility in software design. These classes define common interfaces for derived classes while allowing specific behavior implementations. Mastering polymorphism is essential for developers tackling C++ interview questions, as it demonstrates proficiency in designing scalable, flexible systems.
5. What are virtual destructors, and when are they necessary?
Virtual destructors in C++ ensure proper resource cleanup in polymorphic base classes. When a base class pointer points to a derived class object and gets deleted, the derived class destructor may not execute unless the base class destructor is declared virtual. This leads to incomplete resource cleanup, causing memory leaks. Addressing this issue is essential for developers preparing for C++ interview questions.
For example, consider a base class with a non-virtual destructor. Deleting a derived class object through a base class pointer calls only the base class destructor. Declaring the base class destructor as virtual ensures both base and derived destructors execute correctly. Understanding virtual destructors demonstrates expertise in managing resources in polymorphic scenarios, a vital skill for tackling C++ interview questions.
6. Can you explain multiple inheritance in C++ and how to resolve ambiguity?
Multiple inheritance in C++ allows a class to inherit from multiple base classes. While powerful, it can introduce complexity, such as the diamond problem. This problem occurs when two base classes inherit from a common ancestor, and a derived class inherits from both, creating ambiguity about which ancestor’s attributes to use. Questions about multiple inheritance often appear in C++ interview questions.
C++ offers virtual inheritance to resolve ambiguity, ensuring only one shared instance of the common base class exists. Developers eliminate redundancy and conflicts using the virtual keyword in the base class declaration. Understanding multiple inheritance and the diamond problem equips developers to write clear, maintainable code, a critical skill tested in C++ interview questions.
Memory Management and Performance
Memory management is a cornerstone of advanced C++ interview questions. Topics like dynamic memory allocation, shallow copy vs. deep copy, and optimizing memory space frequently test a developer’s skill in handling performance-critical scenarios.
Here are the three essential memory management and performance concepts to understand:
7. What is the difference between new/delete and malloc/free?
Understanding the difference between new/delete and malloc/free is crucial for tackling C++ interview questions. Both methods manage memory allocation, but they serve distinct purposes. Developers use new/delete in object-oriented programming, while malloc/free caters to low-level memory management needs. These differences impact object lifecycle management and type safety during development.
These are the primary differences between new/delete and malloc/free:
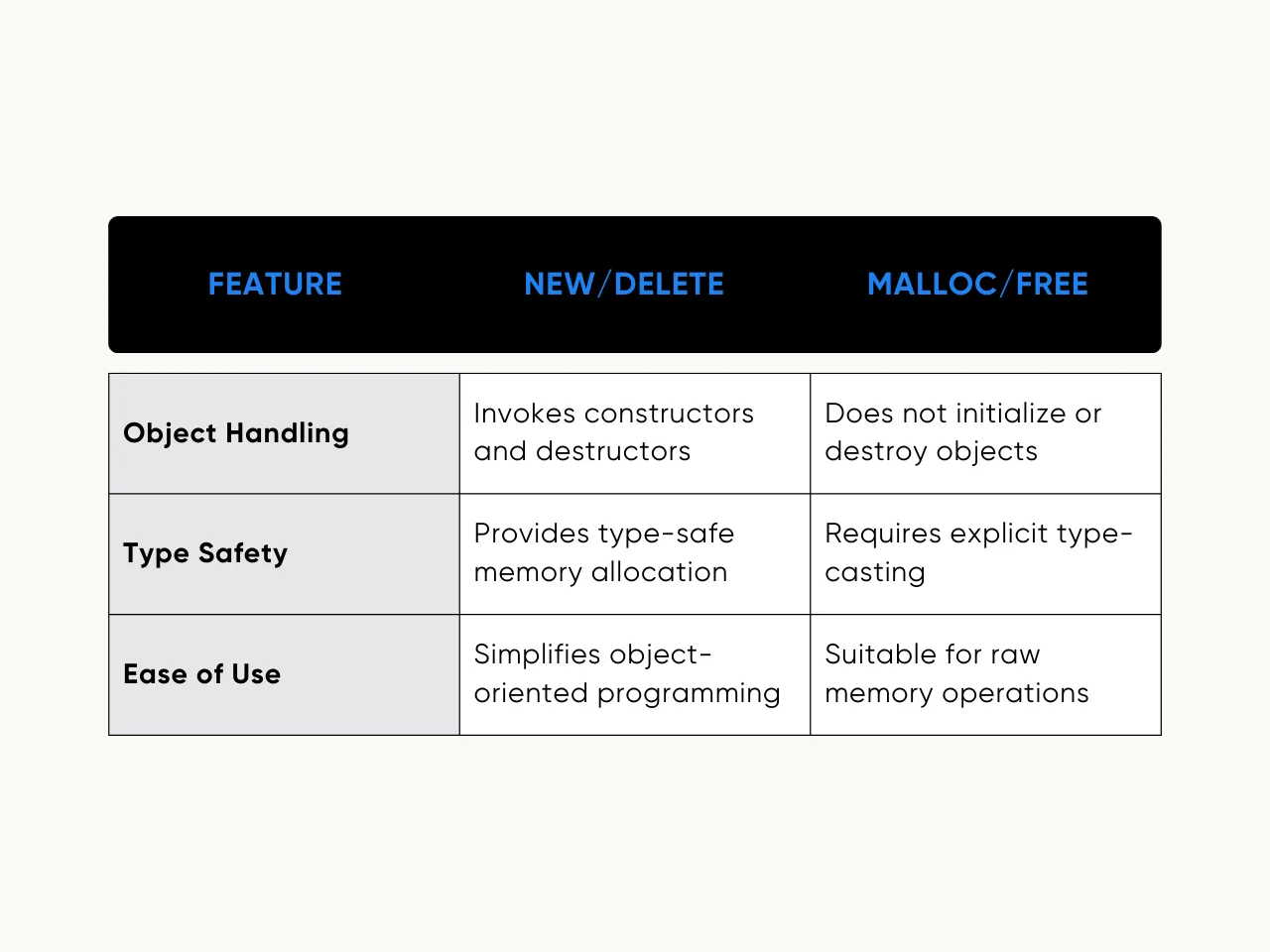
new/delete enables seamless integration with C++ object-oriented features, while malloc/free fits procedural programming needs.
Performance and usage vary with context. Due to its constructor and destructor support, developers rely on new/delete to manage complex objects. In contrast, malloc/free excels in scenarios demanding raw memory control. When preparing for C++ coding interview questions, prioritize understanding these methods and their practical applications. Efficient memory management ensures optimized performance in high-stakes systems.
8. How do you handle memory leaks in C++?
Smart pointers like std::unique_ptr and std::shared_ptr prevent memory leaks in C++ programs. These tools manage the lifecycle of dynamically allocated objects. Smart pointers automatically release memory when the pointer goes out of scope, eliminating manual deallocation.
These are the best coding practices to handle memory leaks effectively:

- RAII (Resource Acquisition Is Initialization): Tie resource management to object lifecycle for automatic cleanup.
- Avoid Raw Pointers: Minimize using raw pointers unless necessary.
- Use std::weak_ptr for Circular References: Break circular dependencies in shared pointers.
- Enable Bounds Checking: Utilize tools and compilers that enforce runtime bounds checking for memory safety.
- Prefer Stack Allocation: Opt for stack-allocated objects over heap allocation whenever possible.
Debugging memory leaks requires precise tools that analyze the program to detect memory mismanagement. A methodical approach to testing and debugging ensures clean and efficient code. Proper practices for handling C++ interview questions related to memory leaks showcase developers' expertise in writing robust programs.
9. What is the role of move semantics and rvalue references in C++11?
Move semantics in C++11 optimize performance by transferring resources instead of copying them. This approach avoids redundant memory allocation and deallocation, making programs faster and more efficient. Rvalue references (&&) enable move semantics, allowing developers to identify temporary objects and transfer their resources directly.
Move semantics are useful for managing dynamic memory, file handles, and other resource-heavy objects. For instance, implementing move constructors and move assignment operators prevents unnecessary deep copies when working with large containers like std::vector. This optimization reduces overhead and improves runtime performance. Mastering move semantics is essential for handling advanced C++ interview questions and showcasing expertise in writing efficient and modern C++ code.
Templates and Generic Programming
Templates are integral to C++ interview questions, showcasing a developer’s ability to write efficient, reusable code. Concepts like variadic templates, template metaprogramming, and SFINAE highlight advanced problem-solving techniques. Mastery in these areas demonstrates coding flexibility.
Here are the three critical template and generic programming concepts to master:
10. What are variadic templates, and how do they work?
Variadic templates in C++ enable functions or classes to accept an arbitrary number of arguments, enhancing flexibility and reusability in code. Introduced in C++11, variadic templates use parameter packs (...) to handle multiple arguments efficiently.
For example, a function to sum arguments can leverage recursion or fold expressions. Recursively unpacking arguments allows the processing of each element, while fold expressions simplify the operation in C++17. Variadic templates are powerful tools for creating generic, reusable code structures and are commonly featured in C++ interview questions, demonstrating expertise in modern C++ programming techniques.
11. What is SFINAE, and how is it applied in C++ templates?
SFINAE (Substitution Failure Is Not An Error) is a C++ feature that allows template overloading based on parameter validity. When a template substitution fails due to incompatible parameters, the compiler does not throw an error but instead skips that template and selects a valid alternative.
For example, std::enable_if can conditionally compile template specializations based on specific traits or conditions. This technique ensures type-safe code and creates highly customizable and efficient templates. SFINAE is a critical concept often appearing in C++ interview questions, showcasing an advanced understanding of C++ template programming.
12. What are the advantages and limitations of template metaprogramming?
Template metaprogramming in C++ allows developers to perform computations during compile time. This technique enables optimized and reusable code structures, improving performance without runtime overhead. Many advanced libraries leverage this feature, making it a critical concept in C++ interview questions.
Here are the advantages of template metaprogramming:
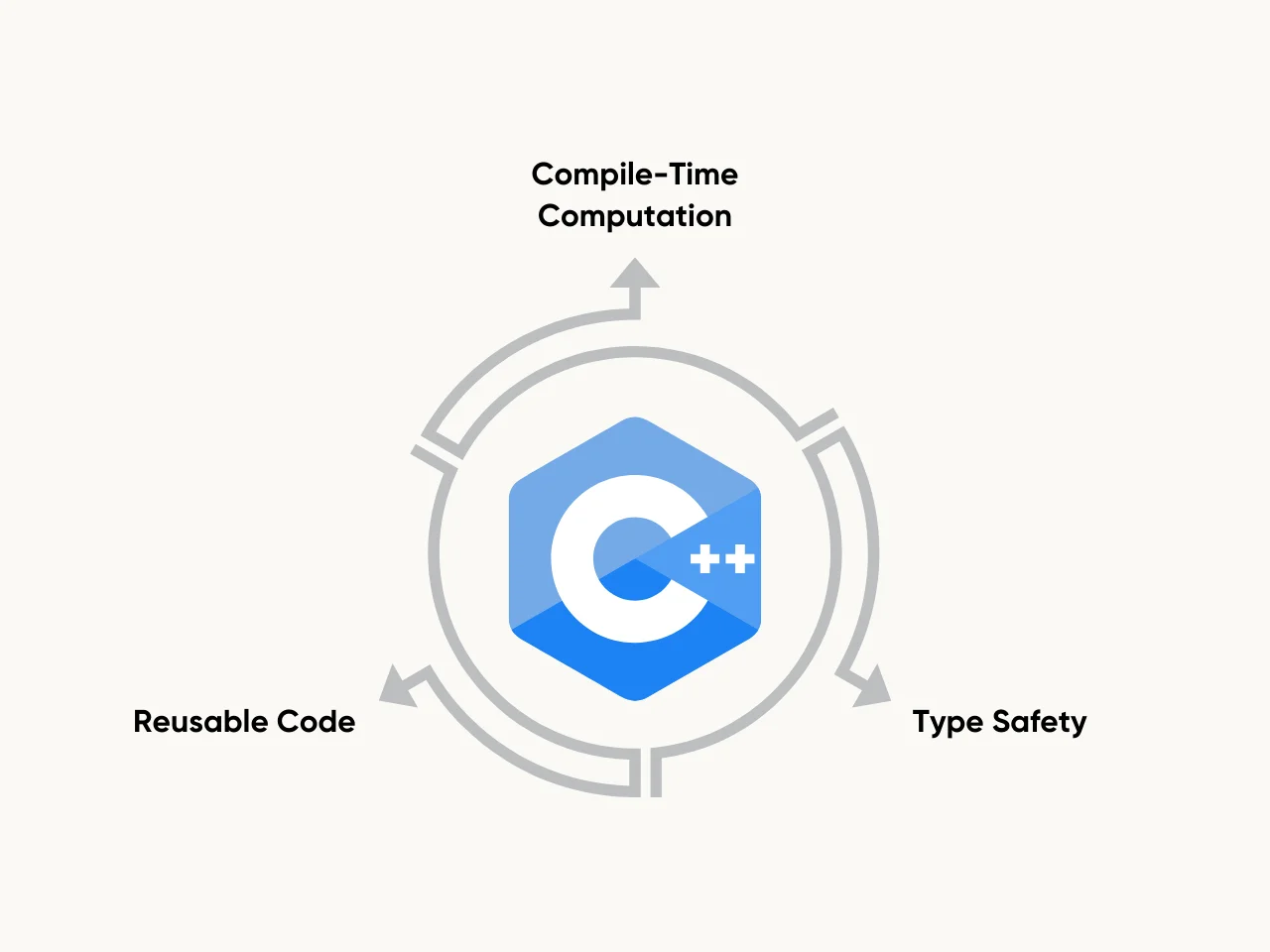
- Compile-Time Computation: This method reduces runtime costs by performing calculations at compile time, improving the program’s execution speed.
- Type Safety: Enforces type checking during compilation, preventing runtime type-related errors. This ensures code reliability.
- Reusable Code: Facilitates the creation of generic and extensible components. This reduces duplication and simplifies code maintenance.
Let's explore the limitations of template metaprogramming:
- Increased Complexity: Code becomes harder to read and maintain, especially for developers unfamiliar with advanced templates.
- Longer Compile Times: Extensive use of templates may significantly increase compilation duration, slowing development cycles.
- Debugging Challenges: Errors in template metaprogramming are often cryptic, making them difficult to trace and fix.
Understanding these aspects of template metaprogramming helps you navigate questions about its implementation and use cases. Mastering this concept can give you an edge in C++ interview questions, showcasing your expertise in advanced programming techniques.
Concurrency and Multithreading
Concurrency topics are common in advanced C++ interview questions and testing expertise in handling threads and synchronization. Key concepts include thread safety, std::atomic, and the C++ memory model, which are crucial for building robust multithreaded applications.
Here are the three fundamental concurrency and multithreading concepts to learn:
13. How do you manage thread safety in C++?
Synchronization tools are critical in managing thread safety when handling C++ interview questions. Developers use mutexes, condition variables, and locks like std::mutex and std::lock_guard to protect class members from simultaneous access. These tools ensure the data members remain consistent across different objects. Employing synchronization prevents errors in legacy code, especially when sharing different types of data between threads. Leveraging the standard template library (STL) enhances efficiency when managing thread-safe tasks.
Avoiding common pitfalls is essential in multithreaded programming. Some potential issues include:

- Deadlocks: Occur when threads wait indefinitely for resources.
- Race Conditions: Arise when threads access class members without proper synchronization.
- Priority Inversion: This happens when high-priority threads wait for lower-priority threads.
- Memory Corruption: Results from modifying a shared memory address without safeguards.
- Starvation: Happens when threads cannot acquire locks, stalling execution.
Implementing a thread-safe queue involves defining an object of a class with synchronized access to its member variables. Use a combination of mutexes and inline functions to protect the block of code managing the queue. Proper exception handling ensures consistent run-time behavior, even when interacting with virtual machines or different data types.
14. What is the role of std::atomic in multithreaded applications?
The std::atomic class in C++ ensures thread-safe operations by providing atomicity. Atomic operations are indivisible, meaning no other thread can interrupt them, which prevents race conditions and guarantees data integrity in multithreaded applications.
A common use case for std::atomic is implementing counters, flags, or shared data structures without locks. For example, an atomic counter can safely track the number of completed tasks in a multithreaded program. Eliminating the need for explicit locks std::atomic reduces overhead and simplifies code. Understanding its role is essential for tackling C++ interview questions, as it highlights expertise in efficient multithreading techniques.
15. How does the C++ memory model ensure consistency across threads?
The C++ memory model ensures thread consistency through well-defined memory access and synchronization rules. It introduces the concept of memory order to control the visibility and sequencing of operations, preventing unexpected behavior in multithreaded programs. The happens-before relationship establishes a predictable execution order between threads, ensuring consistent outcomes.
Synchronization primitives like std::mutex atomic operations with std::memory_order options enforce ordering guarantees. For example, using std::memory_order_release and std::memory_order_acquire ensures proper synchronization between a producer thread writing data and a consumer thread reading it. Mastery of these concepts is vital for addressing C++ interview questions and demonstrating expertise in writing reliable and thread-safe programs.
Key Takeaway
Mastering C++ interview questions is essential for developers aiming to excel in software development. From core concepts like pointers and references to advanced topics such as concurrency and templates, understanding these areas builds confidence during technical interviews. Preparing effectively ensures you showcase problem-solving skills and a deep understanding of the language.
When hiring a C++ developer or enhancing your project’s software quality, consider partnering with experts who bring experience and innovation. A skilled developer ensures efficient code, optimized performance, and a robust project structure. Choosing the right talent sets the foundation for success in complex and performance-critical applications.
Are you ready to tackle C++ interview questions or need help finding the right talent? At Aloa, we guide you through every step of the hiring process. Hire a brilliant C++ developer today and confidently elevate your software development projects.