REST (Representational State Transfer) APIs are fundamental to modern software systems. They provide a lightweight architectural style for designing scalable web service APIs. Mastering REST API principles ensures a seamless user experience and enables efficient communication between client-side applications and rest servers. Understanding concepts like HTTP protocol, request body, and JSON format is essential for those preparing for REST API interview questions.
Aloa offers software outsourcing services that deliver high-quality web and mobile applications. We ensure clients collaborate with top development teams experienced in working with API keys in software applications. Our network of vetted partners, dedicated US-based product owners, and emphasis on transparency led to a portfolio of strong web and mobile applications that have excelled in the market.
Given our expertise in the space, we created this guide to help you review the fundamentals of REST APIs, core components of REST APIs, practical questions on REST API usage, and advanced beginner concepts in REST APIs, so you can be well-prepared for your next REST API interview. At the end of this blog, you’ll be able to confidently tackle common REST API interview questions and excel in your interviews.
Let's get started!
Fundamentals of REST API Interview Questions
Understanding the basics of RESTful web services involves mastering concepts like the uniform interface, standard HTTP methods, and the principles of the REST. From HTTP requests to handling client states efficiently, these fundamentals are key to API proficiency. Here are the REST API interview questions to explore these foundational concepts.
What is a REST API?
A REST API is an application program interface that adheres to REST architecture principles. It uses the Hypertext Transfer Protocol (HTTP) to enable seamless communication between systems. REST APIs deliver resources such as text files, JSON, or XML and remain flexible and scalable. Here are the key characteristics of RESTful APIs:
- Statelessness: Each HTTP request contains all the necessary information for the server to process.
- Uniform Interface: Consistent structure for resources using HTTP request methods like GET, POST, PUT request, and DELETE.
- Client-Server Separation: The client-side (e.g., a web browser) and the API server operate independently.
For example, a weather API provides requested data such as forecasts. Users send a request using an accept header, and the API returns relevant details. The response may include information such as the number of items or client errors using common HTTP status codes like 200 (OK), 404 (Not Found), or 500 (Server Error).
2. What is the Difference Between REST and SOAP?
REST is a flexible, lightweight API design that supports multiple data formats like JSON, making it ideal for web-based applications. SOAP is a protocol with strict standards, commonly used in scenarios requiring high security and reliability, such as in financial and enterprise services.
We’ll break down the key differences between REST and SOAP to help you answer REST API interview questions effectively:
REST and SOAP serve distinct purposes. REST excels in simplicity and flexibility, supporting formats like JSON, making it ideal for modern mobile and web applications. SOAP’s strict structure is better suited for financial transactions, where security and standardization are essential.
What are the HTTP methods used in REST APIs?
HTTP methods are the foundation for communication between clients and servers in REST APIs. These methods define the actions clients can perform on resources to create, retrieve, update, or delete resources, making them essential for efficient API interactions.
Here are some standardized commands used in REST APIs:
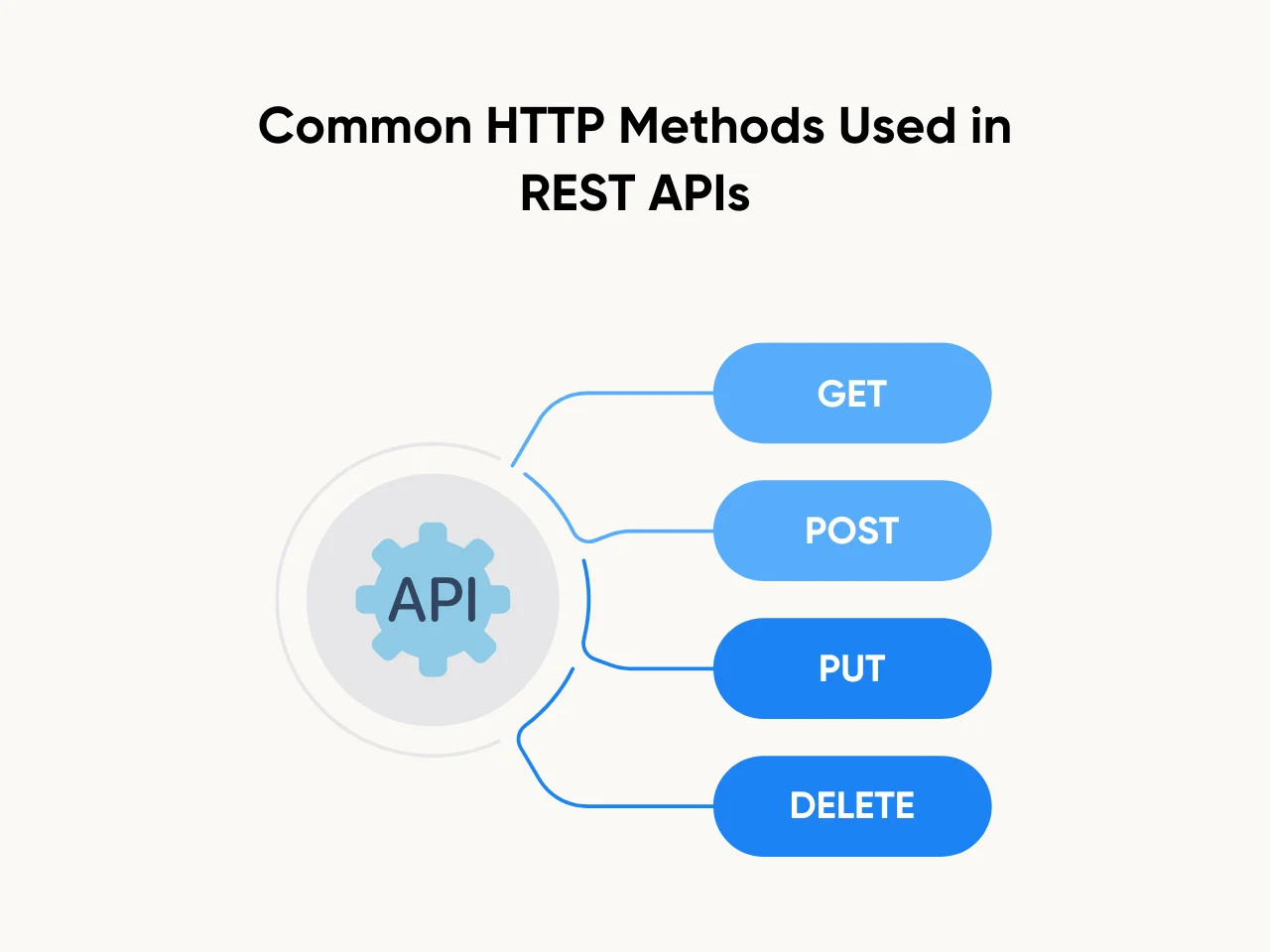
- GET: Retrieves data from a specific resource. It does not modify the resource.
- POST: Creates a new resource on the server. It is commonly used to submit forms or upload data.
- PUT: Updates existing resources. It is idempotent, meaning repeated calls result in the same outcome.
- DELETE: Removes specified resources. Used for tasks like deleting user accounts or files.
Interview Tip: Practice explaining these methods with real-world examples (e.g., “Imagine building a task manager app—how would you design API endpoints using these methods?”).
Core Components of REST APIs
The core components of REST APIs include resources, HTTP methods, URIs, headers, and status codes. Efficient use of query parameters and custom request headers improves the user interface and overall user experience. Here are the REST API interview questions for understanding REST API components.
What are the key principles of REST architecture?
REST architecture offers a strong framework for building scalable web services. It sets principles for structuring, accessing, and managing resources, enabling smooth communication between clients and servers. This makes REST APIs versatile and user-friendly.
Here are the key principles of REST architecture:
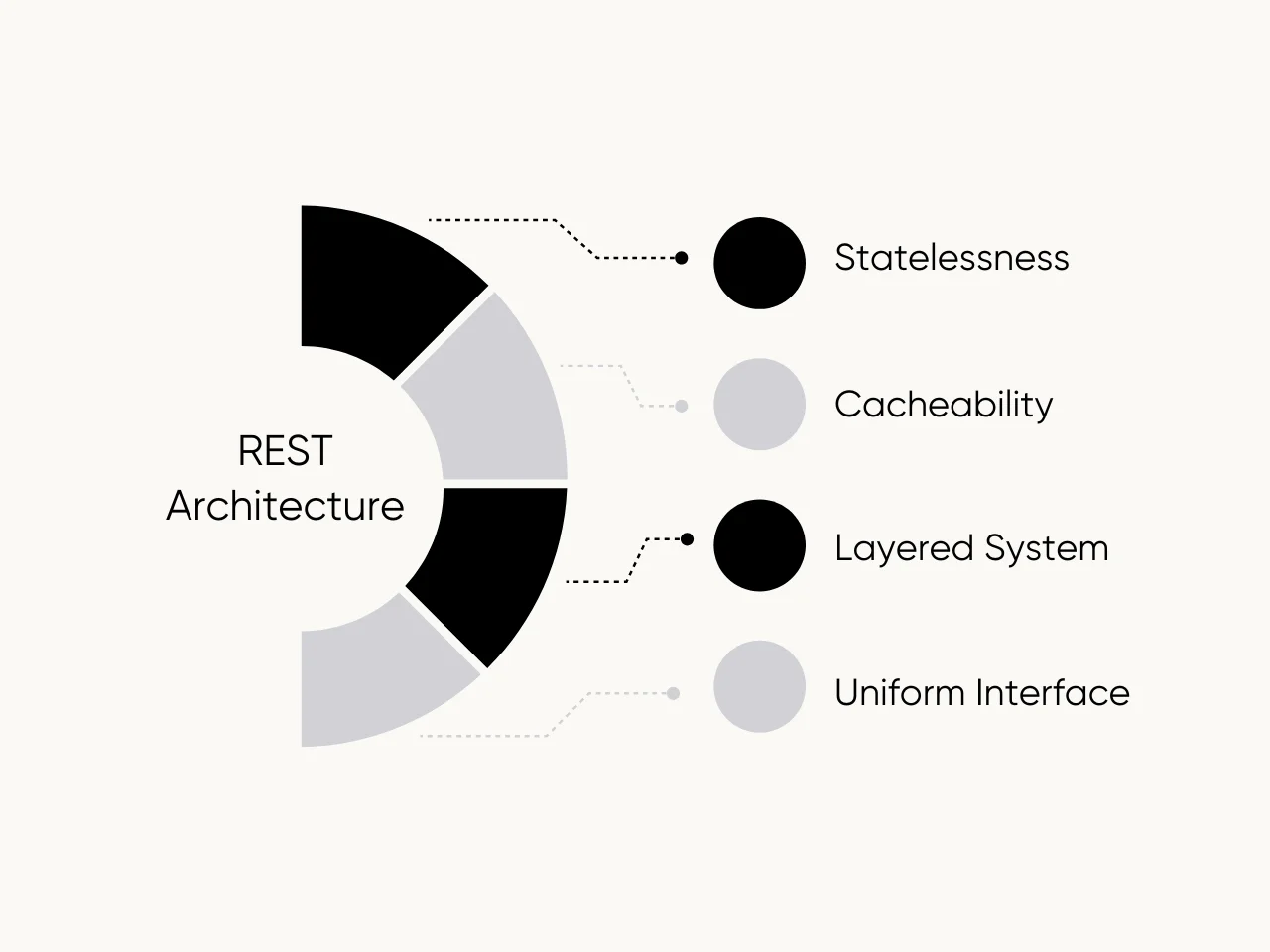
- Statelessness: Every request must carry all the necessary information for the server to process. The server stores no client context between requests.
- Cacheability: Responses should indicate whether they can be cached. This reduces redundant requests and improves performance.
- Layered System: The architecture allows components to operate independently, allowing modular updates without disrupting the entire system.
- Uniform Interface: A consistent structure for resources, such as predictable URIs and HTTP verbs, simplifies client-server interactions.
These principles form the backbone of RESTful APIs, ensuring clarity, efficiency, and scalability. Mastering them helps you create APIs that handle diverse requirements while maintaining simplicity.
What is a resource in REST?
A resource in REST is any object or data entity accessible via a unique URI (Uniform Resource Identifier). It serves as the foundation of RESTful architecture, facilitating interaction between clients and servers. Resources can include files, services, or database records, ensuring structured communication within the system.
For instance, the URI /users/123 refers to a specific user resource in a system, providing unambiguous access to the user’s details. Similarly, /products/45 may represent a specific product in an inventory, allowing clear identification with that item.
What are status codes in REST APIs?
Status codes in REST APIs indicate the outcome of client-server interactions. They give immediate feedback on whether a request succeeded, failed, or encountered an error.
Here are common status codes in REST APIs:
- 200 (OK): The request succeeded, and the server returned the expected data.
- 201 (Created): The server successfully created a new resource.
- 404 (Not Found): The server could not find the requested resource.
- 500 (Internal Server Error): An issue occurred on the server while processing the request.
If you send a successful GET /users/123 request to fetch user details, the server will respond with a 200 (OK) status code. These codes simplify debugging and improve communication between developers.
Practical Questions on REST API Usage
Practical knowledge includes using put methods, handling a patch request, and optimizing the number of requests in a single request. Managing content negotiation, rate limiting, and securing APIs with API keys ensures smooth operations.
Here are the REST API interview questions for applying REST API practices in real-world scenarios:
How do you secure a REST API?
REST API can be secured by implementing authentication (e.g., OAuth 2.0), encrypting data with HTTPS, validating inputs, and setting proper access controls. These measures protect sensitive data, prevent unauthorized access, and ensure reliable service.
Here are the common methods to secure a REST API:

- Use HTTPS to Encrypt Communication: HTTPS encrypts data transmission to prevent interception during requests and responses.
- Implement Authentication: Verify user identities using OAuth or API keys. These methods ensure that only authorized users can access the API.
- Validate Input Data: Validate all incoming data to prevent SQL injection, cross-site scripting, and other vulnerabilities. Input validation enhances the overall security posture.
These methods ensure that only authenticated users can interact with the API, reducing risks and safeguarding data integrity.
What is CORS, and why is it important in REST APIs?
Cross-Origin Resource Sharing (CORS) is a mechanism that controls how resources (such as data, APIs) are shared between different domains. In REST APIs, CORS allows servers to specify which external domains can access their resources, while browsers enforce these rules to block unauthorized requests.
CORS plays a crucial role in the security of browser-based API interactions. For example, an API can use CORS headers to only allow requests from certain trusted domains. This helps ensure that only specified origins can request data.
What is a RESTful URL, and how should it be designed?
A RESTful URL identifies resources in a REST API in a structured way. It reflects the hierarchical organization of resources, ensuring clarity and consistency. Adhering to RESTful design principles makes URLs intuitive, making APIs easier to understand and use.
Key guidelines for designing RESTful URLs include:
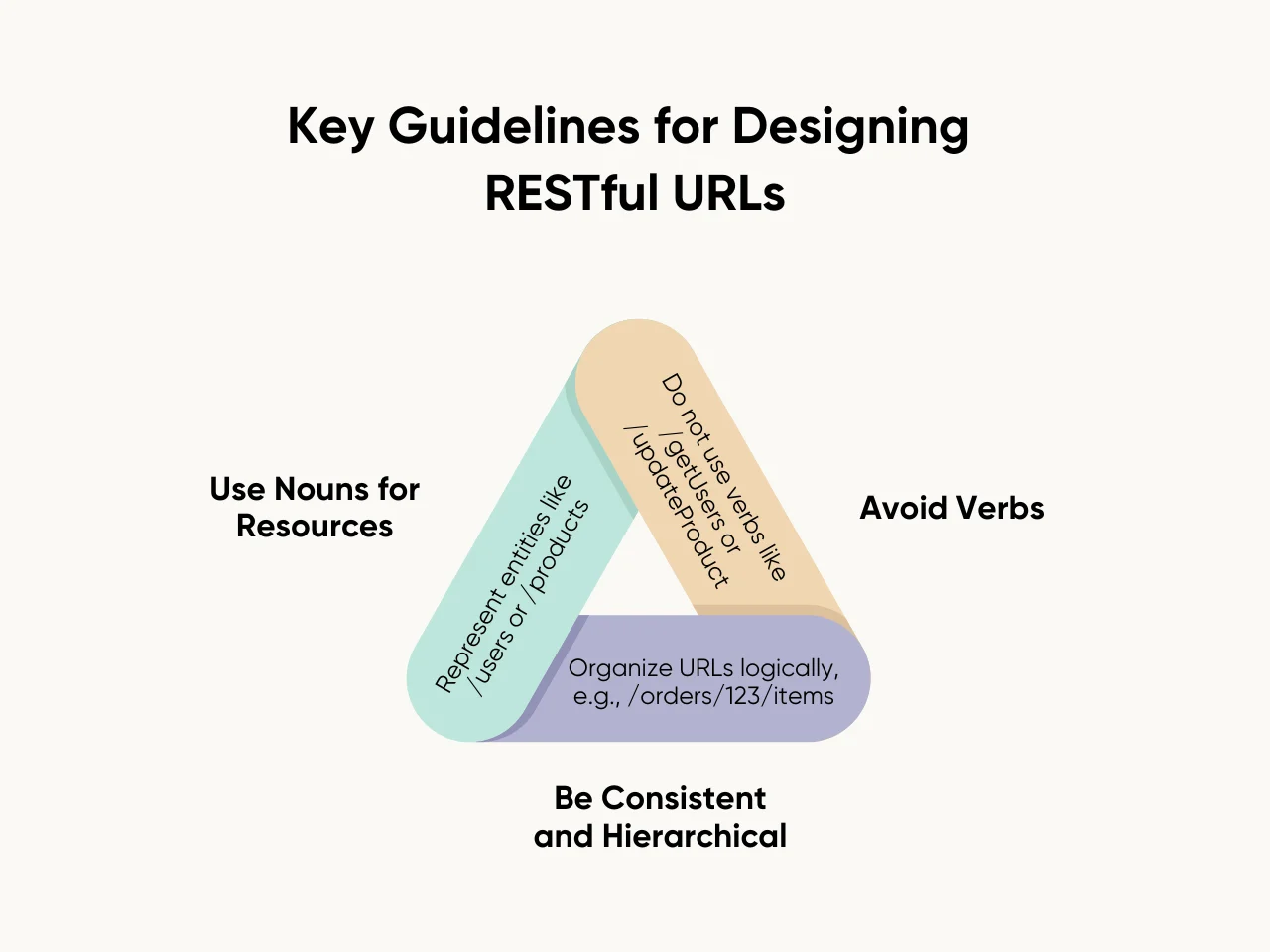
- Use Nouns for Resources: Represent entities like /users or /products instead of actions.
- Avoid Verbs: Do not use verbs such as /getUsers or /updateProduct. The HTTP method already specifies the action.
- Be Consistent and Hierarchical: Organize URLs logically /orders/123/items to retrieve items from a specific order.
For instance, the URL /orders/123/items exemplify a well-structured RESTful design. It identifies the order (123) and related items, making the API functional and user-friendly. This approach ensures clarity in requests and reduces errors in implementation.
Advanced Beginner Concepts in REST APIs
Exploring advanced concepts such as response data, accepting headers, and features of restful web services prepares you for complex challenges. Using the restful architecture and managing client-side errors effectively are essential. Here are the API interview questions for mastering these advanced REST API concepts.
What are query parameters and path parameters?
Query parameters filter or modify API responses and are appended to the URL after a ?. For example, /users?age=25 fetches users aged 25. Path parameters identify specific resources and are embedded in the URL path, such as /users/123, which fetches details for user 123.
When combined, they enable precise and flexible requests, such as /users/123/orders?status=completed. This uses a path parameter (123) to specify the user and a query parameter (status=completed) to filter their orders.
Interview Tip: Practice explaining these parameters by designing an endpoint for a real-world scenario (e.g., “How would you structure a URL to fetch paginated posts from a blog category?”).
What is HATEOAS, and how does it relate to REST APIs?
HATEOAS (Hypermedia As The Engine Of Application State) is a REST API design principle that embeds navigational links within responses. This approach allows clients to discover and interact with related resources dynamically, eliminating the need for hardcoded endpoints by making APIs self-descriptive.
For instance, a /users/123 response might include a link to /users/123/orders, enabling the client to fetch the user data. This approach improves flexibility, scalability, and adherence to REST's uniform interface principle.
How do you handle errors in a REST API?
You can handle errors in a REST API by returning standardized HTTP status codes (e.g., 400 bad requests or 404 not found) and detailed error messages. Clear error responses help users diagnose issues quickly, which improves the overall API experience.
Here are the key methods to handle errors in a REST API:
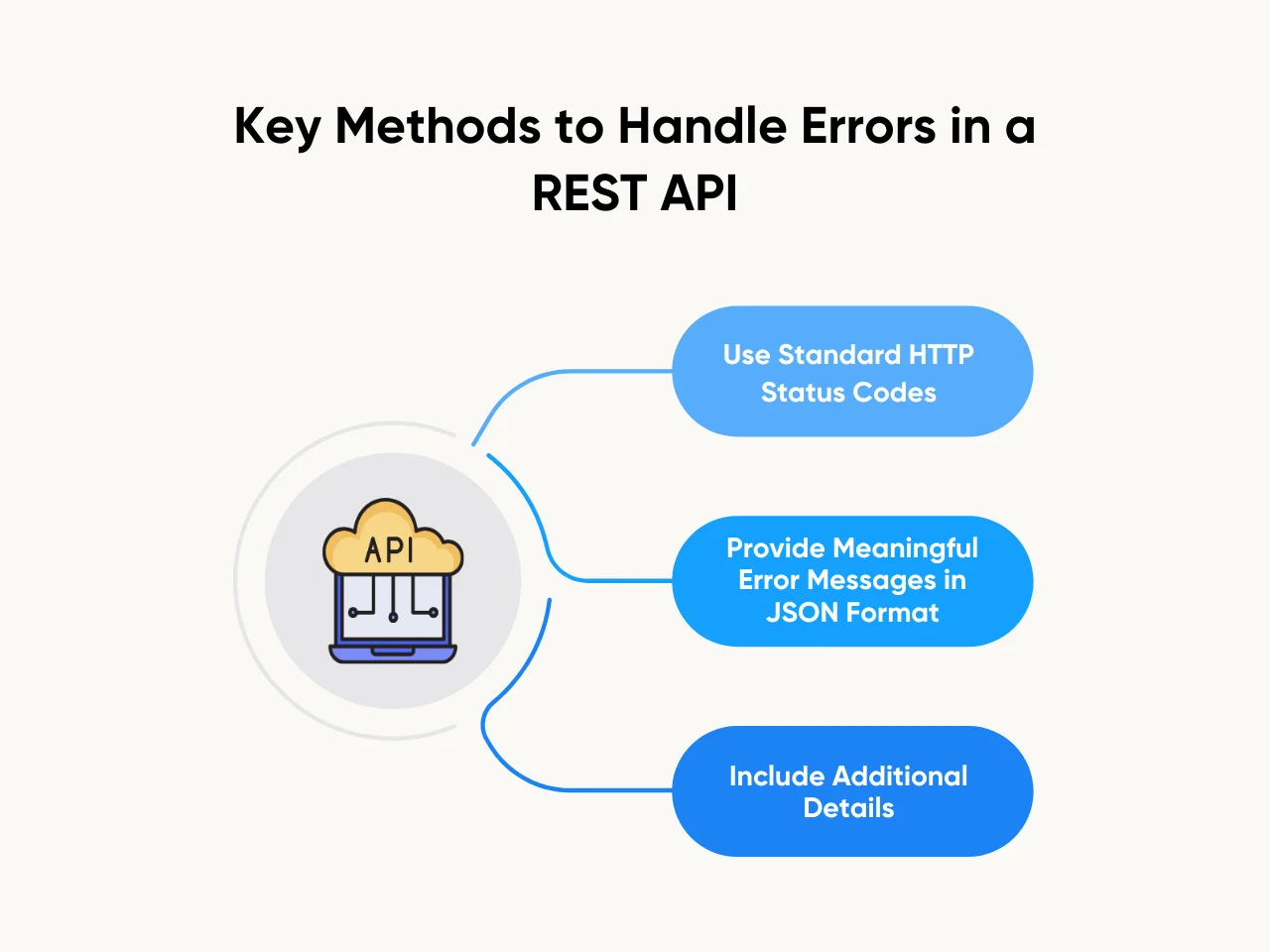
- Use Standard HTTP Status Codes: Indicate the request outcome using status codes like 404 (Not Found) or 500 (Internal Server Error).
- Provide Human-Readable Messages: Deliver descriptive messages that explain the issue.
- Include Additional Details: Enhance error responses with additional details or timestamps to help with debugging.
Effective error handling makes debugging much more efficient.
Interview Tip: Highlight how these methods improve API usability and make client integration easier to show your expertise in building user-friendly APIs.
Other Questions to Help You Prepare for REST API Interviews
To prepare for your interview, you should build a strong understanding of web API services and best practices. Here are some additional REST API interview questions:
What tools would you use to test REST APIs?
Testing tools allow you to validate endpoints, identify bugs, and optimize API performance. Here are some common tools for testing REST APIs:
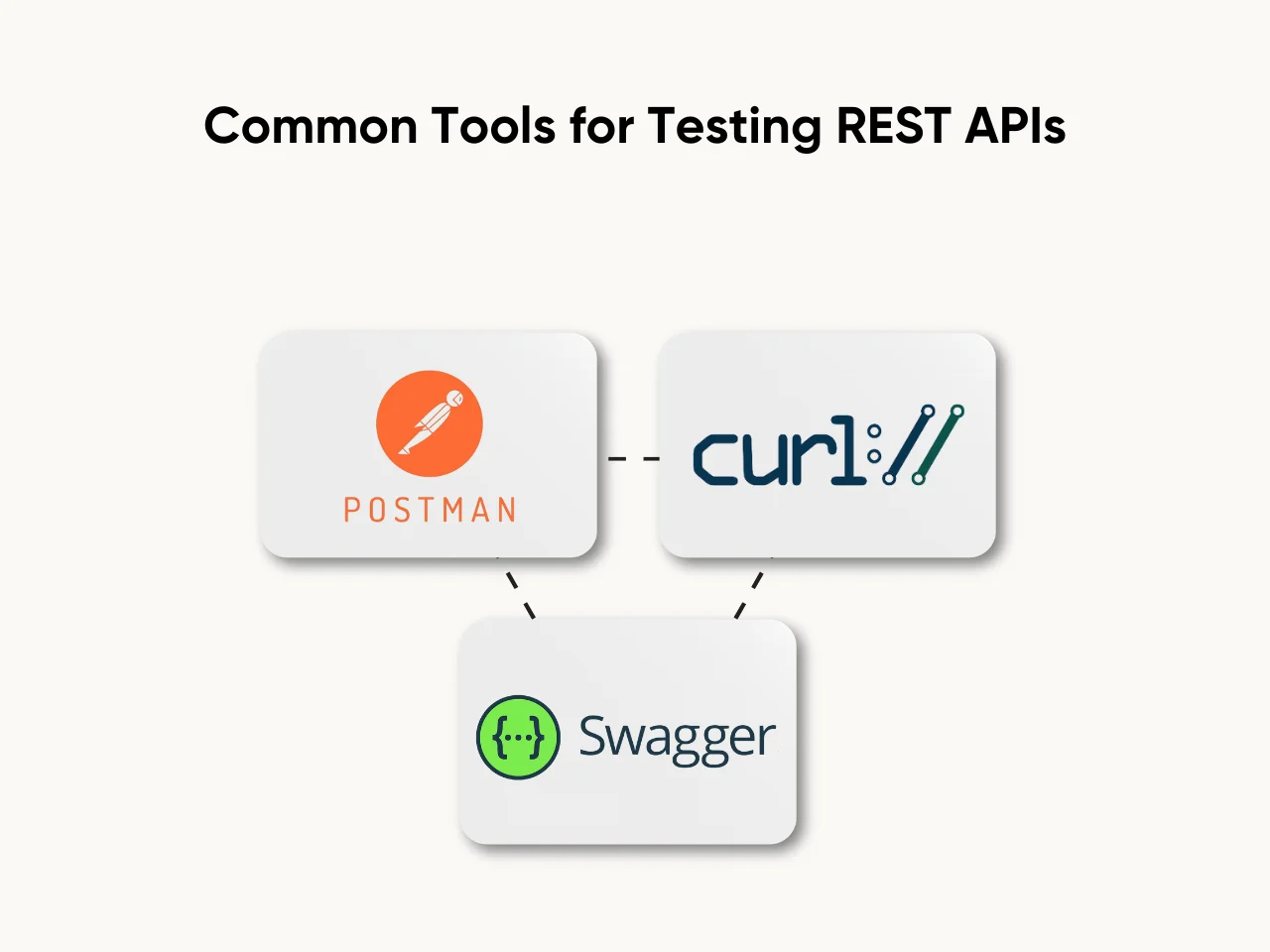
- Postman: A user-friendly tool for creating, sending, and analyzing API requests. It supports various HTTP methods and offers features like automation and collaboration.
- cURL: A command-line tool used for making HTTP requests. You can perform GET, POST, and other methods directly from the terminal.
- Swagger UI: Visualizes and tets APIs while reviewing their documentation. It simplifies endpoint testing.
Interview Tip: Practice explaining how you’d test a specific scenario (e.g., “How would you validate xyz in a GET /orders endpoint using Postman?”).
Can you describe your prior experience with REST APIs?
When addressing REST API interview questions, you should focus on real projects you’ve worked on. Share your experience designing or implementing APIs and focus on their purpose and functionality. Highlight tasks you’ve done such as integrating APIs into applications, managing data retrieval, or creating endpoints for seamless system communication.
For example, you can mention designing an e-commerce REST API with secure authentication and scalable architecture. Briefly explain how you solved challenges, like securing data with OAuth or boosting performance using caching. Show how these solutions improved efficiency and user experience. Make sure your thoughts are well-organized when demonstrating your expertise and problem-solving skills.
Key Takeaway
These REST API interview questions will help you prepare for technical discussions and stand out in interviews. Make sure you review the core principles of REST APIs, including HTTP methods, status codes, and design best practices. If you’re unfamiliar with any of these, be sure to take on additional projects and learn to handle related tasks effectively in real-world scenarios.
Designing an API requires attention to details such as resource naming, security, and error handling. Through consistent practice over time, even beginners can develop a solid foundation to design scalable and efficient APIs for various applications.
Are you ready to enhance your knowledge of REST API interview questions and boost your confidence in tackling API-related challenges? At Aloa, we provide expert guidance and tools tailored to your software development needs. With a transparent process and access to industry-leading solutions, we ensure your projects meet the highest standards. Connect with us and start your white-glove outsourcing experience.