Java, a cornerstone of modern programming, relies heavily on APIs to streamline software development processes. One such crucial API is the Java String API, which handles strings within Java programs. The String class facilitates the manipulation and management of string objects, representing sequences of characters. It offers versatile functionality, empowering Java developers to work efficiently with strings in their Java applications.
Aloa, an expert in software outsourcing, recognizes the pivotal role of String Class in Java in crafting efficient solutions. Leveraging our proficiency and industry experience, we offer comprehensive services tailored to meet diverse client needs. Our adept team harnesses the power of the String manipulation in Java to optimize performance and streamline development processes, ensuring exceptional outcomes for every project.
Based on our experience in Java programs, we've put together this guide that delves into the Java String API. Here, we’ll explore its features and the benefits of leveraging Java String functions. Afterward, you will gain a comprehensive understanding of managing strings in Java, empowering you to write more efficient and effective Java applications.
Let's dive in!
What is a Java String API?

Java String API is a comprehensive set of tools and methods provided by the Java language for manipulating strings, which are sequences of characters. It includes functions for string concatenation, handling mutable strings, creating substrings, and converting between different representations like byte arrays or character sequences.
Here are the five use cases of Java String API:
- String Concatenation: Uses the "+" operator or StringBuilder/StringBuffer class to combine multiple strings efficiently.
- Substring Extraction: Retrieves a substring of a string based on a specified index or range.
- Character Manipulation: Accesses and modifies individual characters of a string.
- Regular Expression Matching: Searches and manipulates strings based on given patterns.
- Case Conversion: Converts strings to uppercase or lowercase as required.
Java String API provides exceptional support for various operations like finding a character's first or last occurrence, counting occurrences, handling whitespace, and more. It is a fundamental component in Java programming, facilitating efficient string handling and manipulation tasks.
How to Build a Java String API
Building a comprehensive Java String API involves meticulous planning and implementation. We can create a robust toolset for string manipulation in Java applications by carefully crafting each component. Here are five essential steps to construct the Java String API, ensuring versatility and efficiency in handling string operations:
Step 1: Define String Representation
To begin building the Java String API, it's essential to establish a robust representation for strings. This involves creating a dedicated class, such as 'MyString,’ which encapsulates the concept of strings as a sequence of characters. This class's methods should be implemented to handle various aspects of string manipulation.
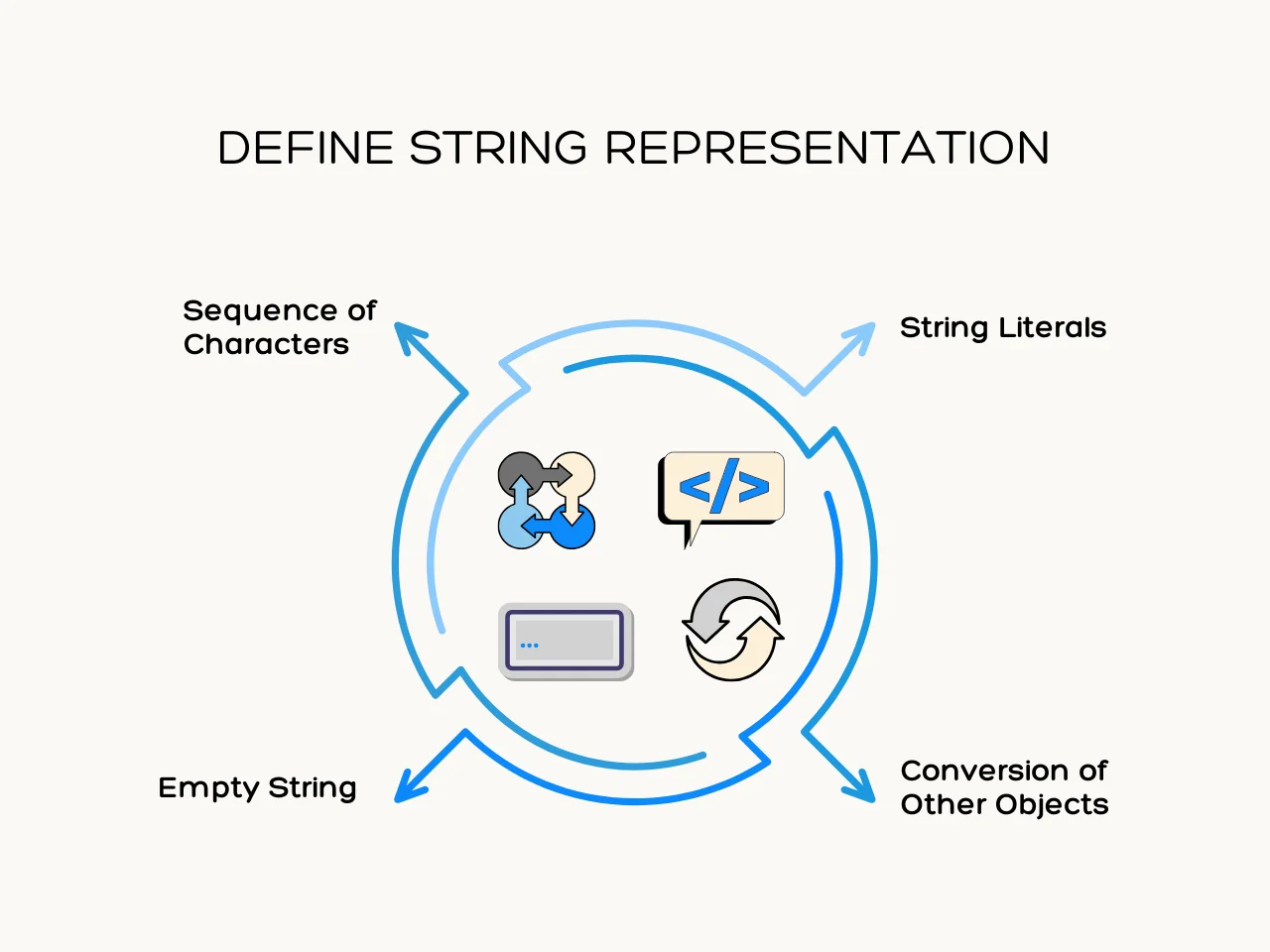
Here are the key things to consider:
- Sequence of Characters: The 'MyString' class should encapsulate the idea of a sequence of characters, providing methods to manipulate and access these characters effectively.
- Empty String: Considerations should be made to handle empty strings, ensuring the API can accommodate and manipulate them appropriately.
- String Literals: Implement methods to work with string literals, allowing for easy instantiation and manipulation of strings within the API.
- Conversion of Other Objects: Enable the conversion of other objects, such as integers or floats, into string representations within the ‘MyString’ class.
Defining a solid foundation for string representation, the subsequent steps in building the Java String API can build upon this framework. This ensures versatility and functionality in string manipulation tasks, including those relevant to Spring Boot development.
Step 2: Implement String Concatenation
To ensure effective string manipulation within the Java String API, it's crucial to implement robust string concatenation methods. This step integrates functionalities to seamlessly concatenate strings, utilizing the + operator or the ‘StringBuilder’ class.
Follow these steps to implement string concatenation:
- Utilize the + operator for simple concatenation operations where string immutability isn't a concern.
- Employ the 'StringBuilder' class for more complex concatenation scenarios, as it offers superior performance by efficiently appending characters to an existing sequence.
- Ensure that the concatenation process preserves the integrity of the original strings and accurately combines them without altering their content.
- Optimize the concatenation algorithm to handle various scenarios, including concatenating string literals, empty strings, or strings with different lengths.
Implementing robust string concatenation methods can offer developers efficient tools to manipulate strings effectively, catering to diverse application requirements and enhancing overall programming productivity.
Step 3: Handle Unicode and Subarrays
Addressing the complexities of text data requires meticulous attention to Unicode and manipulating character subarrays. This stage ensures the API can handle various text-processing tasks precisely and flexibly. Here are the things to consider when handling Unicode and Subarrays:

- Unicode Support: Implement methods that efficiently manage Unicode code points, recognizing the diversity of text encountered in global applications. This involves handling the full range of Unicode code units and ensuring compatibility across different languages and symbols.
- Character Subarrays: Enable the extraction of specific subarrays from the string, utilizing specified indices or ranges. This feature is vital for operations requiring a subset of the string, whether for analysis, modification, or extraction purposes.
- Comprehensive String Functions: Introduce functions to find substrings, including the string of a specified character's last or first occurrence. This capability is indispensable for text parsing and manipulation tasks.
- Flexible Buffer and Array Handling: Facilitate using string buffer arguments and the conversion of character arrays to strings. This includes creating strings from specified subarrays of bytes or character arrays, catering to a broad spectrum of text processing needs.
- Advanced Text Features: Address the nuances of text representation, such as managing strings with specified suffixes, accommodating case differences, and handling text in the default locale. These considerations ensure the API is versatile and adaptable to various text-processing scenarios.
- Efficient Data Management: Incorporate methods to process additional information like the contents of a subarray or the count argument, enhancing the API's ability to handle detailed text data efficiently.
The capability to handle Unicode and character subarrays forms a foundation for robust text processing. This step expands the API's utility and ensures its relevance in handling the complexities of modern text data, catering to a wide range of application needs.
Step 4: Support Character Operations
To enhance its functionality, focusing on character operations is essential. This step is crucial to enhance the API's ability to manage and manipulate characters within strings effectively. This phase involves several key considerations:
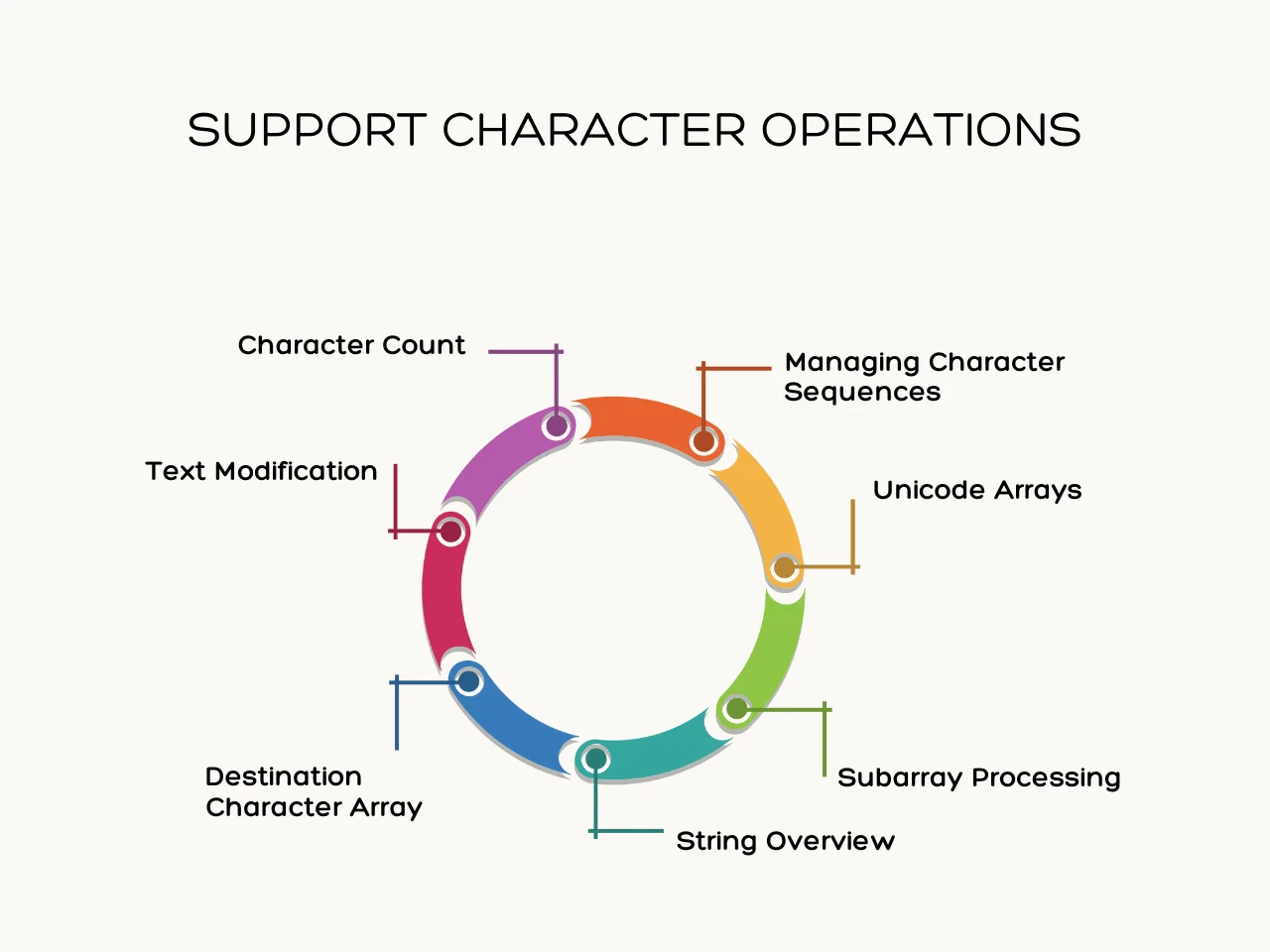
- Identifying Character Occurrences: Implement methods to pinpoint specific characters' first and last occurrences. This allows for precise string analysis and manipulation based on character presence.
- Managing Character Sequences: Equip the API to adeptly handle empty character sequences, ensuring robustness when dealing with strings that may not contain visible characters.
- Character Array and Unicode Support: Facilitate operations on char arrays and subarrays, including those represented as Unicode code point arrays. This encompasses converting these arrays into strings, catering to diverse text range requirements.
- Subarray Processing: Introduce functionalities to process specified subarrays of bytes, enabling advanced manipulation and representation of string data.
- Comprehensive String Representation: Ensure the class string can offer string representations for various data types, including char array, char, and boolean arguments. This versatility is crucial for a fully featured String API.
- Destination Character Array: Allow methods to directly affect the destination character array, providing a direct pathway for modified string data.
- Adjusting Text Range and Code Points: Support operations that adjust the text range within a string or manipulate codePointOffset points, facilitating complex text processing tasks.
Comprehensive character operations significantly elevate its utility. Implementing these functionalities, developers gain a powerful tool for string manipulation, covering a broad spectrum of use cases from simple character searches to complex text transformations.
Step 5: Manage Additional Features
Enhancing the API with additional functionalities significantly improves its versatility and usability across various applications. Building this API focuses on integrating features, extending its capabilities beyond simple string manipulation. This stage is crucial for introducing a range of functionalities that cater to diverse programming needs:
- Handling Space Characters and White Spaces: Implement methods to manage space characters effectively. This includes detecting and manipulating white spaces within strings, which is vital for text processing and user inputs.
- Counting Characters: Introduce functionality to count characters within a string, including those in a specified subarray of bytes or a subarray of the Unicode code point array argument. This feature is essential for detailed text analysis.
- Managing Case Differences: Equip the API with capabilities to handle case differences within strings. This enhancement allows for more flexible string comparisons and manipulations, accommodating the need for case-insensitive operations.
- Support for String Representations of Various Data Types: Broaden the API’s utility by supporting string representations of different data types, including char arrays, boolean arguments, and integers. This involves implementing methods for the string representation of these arguments: the char, char array, and the boolean.
- Identifying Specific Characters or Substrings: Develop methods to locate the string of the last and first occurrence of a specified character within the original string. This also extends to handling specified strings and the contents of the subarray, enhancing the API’s search and manipulation capabilities.
Enhancing the Java String API with these additional features makes it a more powerful tool for developers. With effective management of white spaces, case differences, and the representation of various data types, the API becomes significantly more versatile and capable of handling complex string manipulation tasks.
Key Features of Java String API
The Java String API is a powerhouse packed with features essential for manipulating sequences of characters efficiently. Understanding these ten key features will enhance your programming prowess in Java:

- Immutable Character Strings: Once you create a string in Java, you can't alter it. This immutability ensures reliability and thread safety across your applications.
- String Literal Pool: Java optimizes memory usage by storing identical string literals in a single location. This mechanism reduces memory overhead and speeds up string processing.
- String Concatenation Operator (+): Java allows easy concatenation of strings using the '+' operator, making constructing new strings from existing ones straightforward.
- StringBuilder and Buffer: For scenarios demanding mutable strings, Java offers StringBuilder and StringBuffer, enabling efficient modification of character strings without creating multiple string instances.
- Character and Substring Searches: The API provides methods to find the string of the first or last occurrence of a specified character or substring, enhancing search capabilities within strings.
- Conversion Utilities: String manipulation in Java supports the conversion of other objects into strings, facilitating interaction between strings and various data types through methods like toString() for objects or valueOf() for primitive data types.
- Regular Expressions and Splitting: It offers robust pattern matching and text manipulation capabilities, including splitting strings based on regex patterns, matching sequences, and replacing substrings.
- Unicode Support: The API fully supports Unicode, allowing the representation and manipulation of various characters beyond ASCII, including operations on Unicode code points and units.
- Trimming and Case Conversion: Methods for trimming white space from the beginning and end of strings and converting cases considering locale ensures flexibility in handling user input and data.
- Length and CharAt: Developers can quickly ascertain the number of characters in a string and access individual characters, aiding in detailed text analysis and manipulation.
Benefits of Java String API in Software Development
The Java String API offers a powerful toolkit for handling text data in software development. This API's versatility allows developers to manipulate character sequences efficiently, from basic string manipulation to complex text processing tasks. Here are five key benefits:

Efficient String Manipulation
With the Java String functions, developers can easily create new strings, modify existing ones, and perform operations like string concatenation. The API provides methods for converting entire strings or substrings to new character arrays, enabling quick modifications and searches within strings. For example, finding the substring of this string or generating a copy of a string becomes straightforward, enhancing code readability and maintainability.
Robust Character Handling
Character handling is a cornerstone of text processing. The Java String API excels here by offering direct methods to work with individual characters of the sequence, whether assessing the string of the first occurrence of a specified character or manipulating Unicode code points. Developers can also easily convert specified subarrays of bytes into strings, catering to a wide range of applications, from data parsing to internationalization.
Advanced Text Processing Features
The API's support for advanced text processing, such as matching strings against given regular expressions or comparing string regions, underscores its utility in developing sophisticated software solutions. Performing operations like case conversion (upper case or lower case) based on the rules of the given locale or splitting strings using the specified array of bytes showcases the API's flexibility and depth.
Seamless Integration with Java Ecosystem
String Class in Java integrates with other Java classes, like StringBuilder and StringBuffer, which enhances its functionality. This special support allows for efficient string concatenation and modifications without the overhead of creating multiple string objects. It ensures that operations like appending the string representation of various objects or merging CharSequence elements are optimized for performance.
Convenience and Usability
Creating a new string, whether from a sequence of char values, a character array, or even converting other objects into strings, is made user-friendly with the Java String API. It supports creating an empty or newly created string object from an array, making it a versatile tool for software development. Furthermore, functions that consider locale-specific rules and string representation of the object argument enhance the API's usability across different regions and applications.
Key Takeaway
The Java String API stands as a cornerstone for developers aiming to harness the full potential of Java. It provides a robust framework for manipulating strings, which is crucial for basic and advanced programming tasks. Understanding the Java String functions enables precise control over strings, from finding the string of the last occurrence (specified character) to efficiently managing the string of the first occurrence of a character.
With Java String API, it makes converting data types into their string representation seamlessly. Whether it's a representation of the char or the boolean argument, this API simplifies the process, ensuring developers can focus on logic rather than getting bogged down by data type conversions. Additionally, the ease of working with character array arguments highlights the API’s flexibility, catering to various programming needs.
Sign up for Aloa's email list to deepen your understanding and stay updated with Java developments, ensuring you remain at the forefront of technological advancements. We offer insights and updates directly to your inbox, keeping you informed and ahead in your development journey.