Senior developer interviews demand technical expertise and precision. Employers look for candidates who deeply understand C# interview questions and can solve complex problems efficiently. The ability to navigate concepts like value type, reference type, garbage collection, and exception handling often separates top-tier developers from the rest. Mastering these concepts ensures readiness to tackle challenges related to programming language fundamentals and practical scenarios.
At Aloa, we leverage expertise in software development to guide teams toward success. Our network of vetted partners ensures access to cutting-edge programming language tools and frameworks. We simplify complex data structure projects, ensuring seamless integration with SQL servers and other data sources. Clients benefit from streamlined processes for tackling challenges involving unmanaged code, managed code, or asynchronous programming.
Based on our proven strategies, we've created this guide covering essential C# interview questions for senior roles. By the end, you'll have a roadmap for answering senior-level questions and elevating your years of experience with C# programming to the next level. Start preparing now for senior-level C# interview questions success!
Let's get started!
Advanced Technical C# Interview Questions
Senior developer roles require a deep understanding of C# programming concepts and problem-solving skills. Mastering advanced topics like memory management, asynchronous programming, and runtime environments can set you apart. Here are five essential questions to help you prepare for technical challenges in C# interviews and demonstrate expertise in software development.
1. What is the difference between abstract classes and interfaces in C#?
Abstract classes and interfaces in C# are blueprints for creating objects but differ in functionality and use cases. Abstract classes allow the definition of methods with or without implementation, providing a mix of abstract and non-abstract methods. Interfaces, on the other hand, strictly define contracts without implementation (before C# 8.0). Both are essential tools when designing reusable and modular code.
These are the key differences:
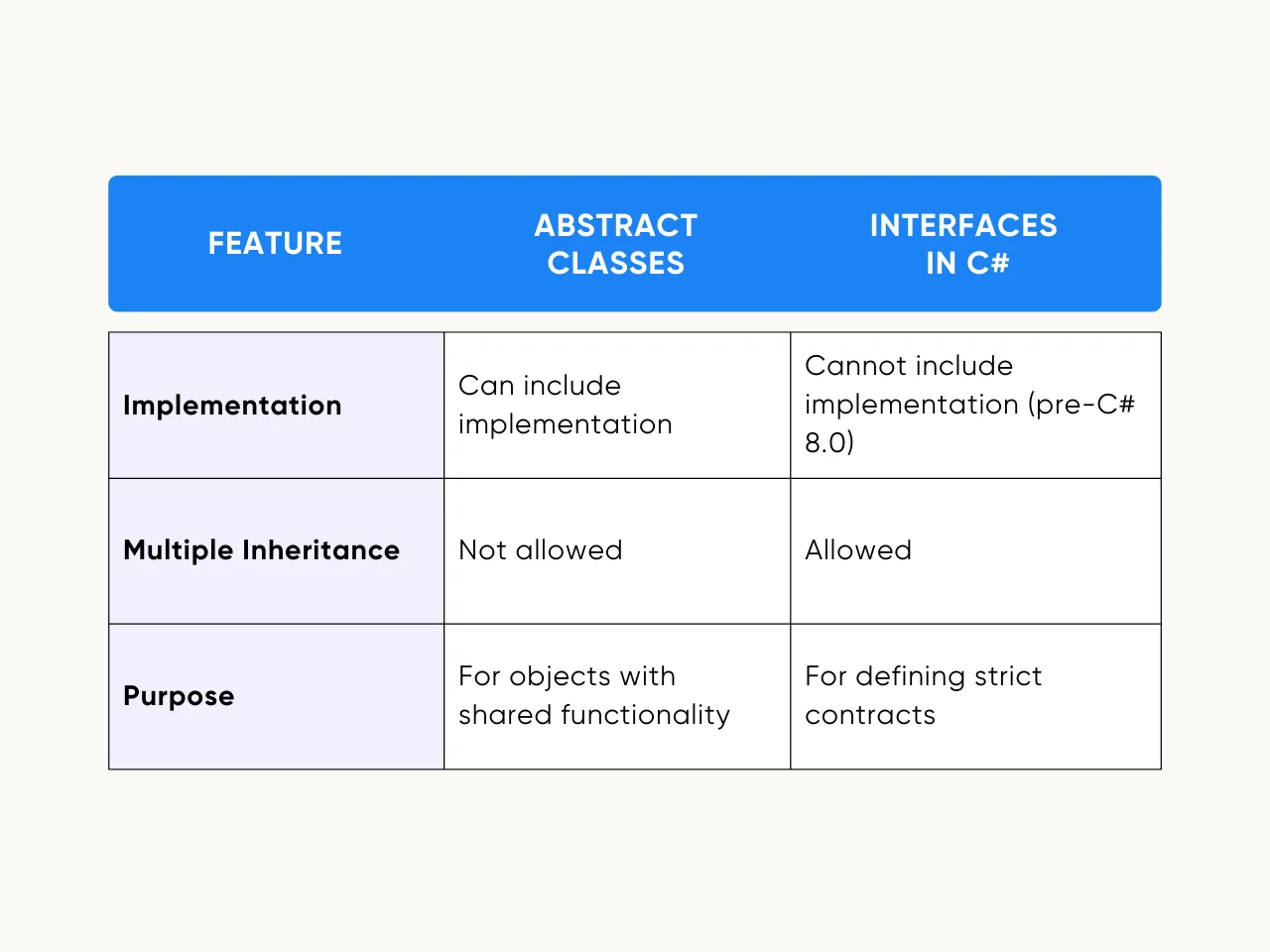
Developers choose between abstract classes and interfaces based on project needs. Use abstract classes when creating objects with shared behavior and interfaces when multiple inheritance or flexible contracts are required. For example, an abstract Shape class could define shared methods like Draw(), while an IDrawable interface ensures consistency across unrelated objects, such as Shape and UIControl.
2. How does garbage collection work in .NET, and how can you optimize it?
The garbage collector in .NET automatically reclaims memory space allocated to unused objects, ensuring efficient memory management. It identifies instances of a class, static objects, and other resources no longer referenced and frees up resources, eliminating manual file handling or stream bytes cleanups. This process minimizes memory leaks and maintains application performance.
.NET’s GC organizes memory into three generations:
- Gen 0: Holds short-lived objects like temporary variables. The GC frequently cleans this generation.
- Gen 1: Serves as a buffer between short-lived and long-lived objects.
- Gen 2: Stores long-lived objects, such as string class data or special methods within partial classes, requiring infrequent cleanup.
Enhance performance by reusing objects through object pooling and minimizing unnecessary allocations to reduce GC triggers. Manage large objects using jagged arrays or arrays of arrays for efficient memory use. Careful use of member functions and foreach loops optimizes resources and ensures scalability.
3. Can you explain covariance and contravariance in C#?
Covariance allows assignment compatibility for output types, enabling derived types to replace base class types in generic collections. For instance, in IEnumerable<T>, a derived class like IEnumerable<string> can be assigned to IEnumerable<object> because string derives from object. This is particularly useful when iterating through data collections with a foreach loop.
Contravariance, on the other hand, permits broader input types, allowing flexibility for member functions that accept parameters. For example, in Func<in T, out TResult>, contravariance applies to T, enabling Func<object, string> to be assigned to Func<string, string>. These principles optimize reuse while maintaining type safety in instances of a class.
4. What are extension methods in C#? How are they useful?
Extension methods enable developers to add new functionality to existing types without using inheritance or modifying the original class object. These methods appear as part of the base class but are defined in a static class and use the this keyword as the first parameter to specify the extended type. Extension methods maintain clean and modular code, especially when working with third-party libraries where altering the source code isn’t possible.
For example, an extension method ToCsv() can transform a collection into a CSV string. Applied to a list of objects, it iterates using a foreach loop, formats value pairs into a stream of bytes, and returns a well-structured CSV string. This allows reuse without creating a derived class or duplicating code.
5. How does asynchronous programming work in C#?
Asynchronous programming in C# allows efficient handling of tasks without blocking execution. Using the async and await keywords, developers can manage tasks in a non-blocking manner. The Task and Task<T> classes handle asynchronous operations, distinguishing between CPU-bound tasks (requiring computation) and I/O-bound tasks (waiting for input or output operations). Understanding these is crucial for mastering C# interview questions, as it demonstrates expertise in optimizing code execution.
Common pitfalls in asynchronous programming and how to avoid them:
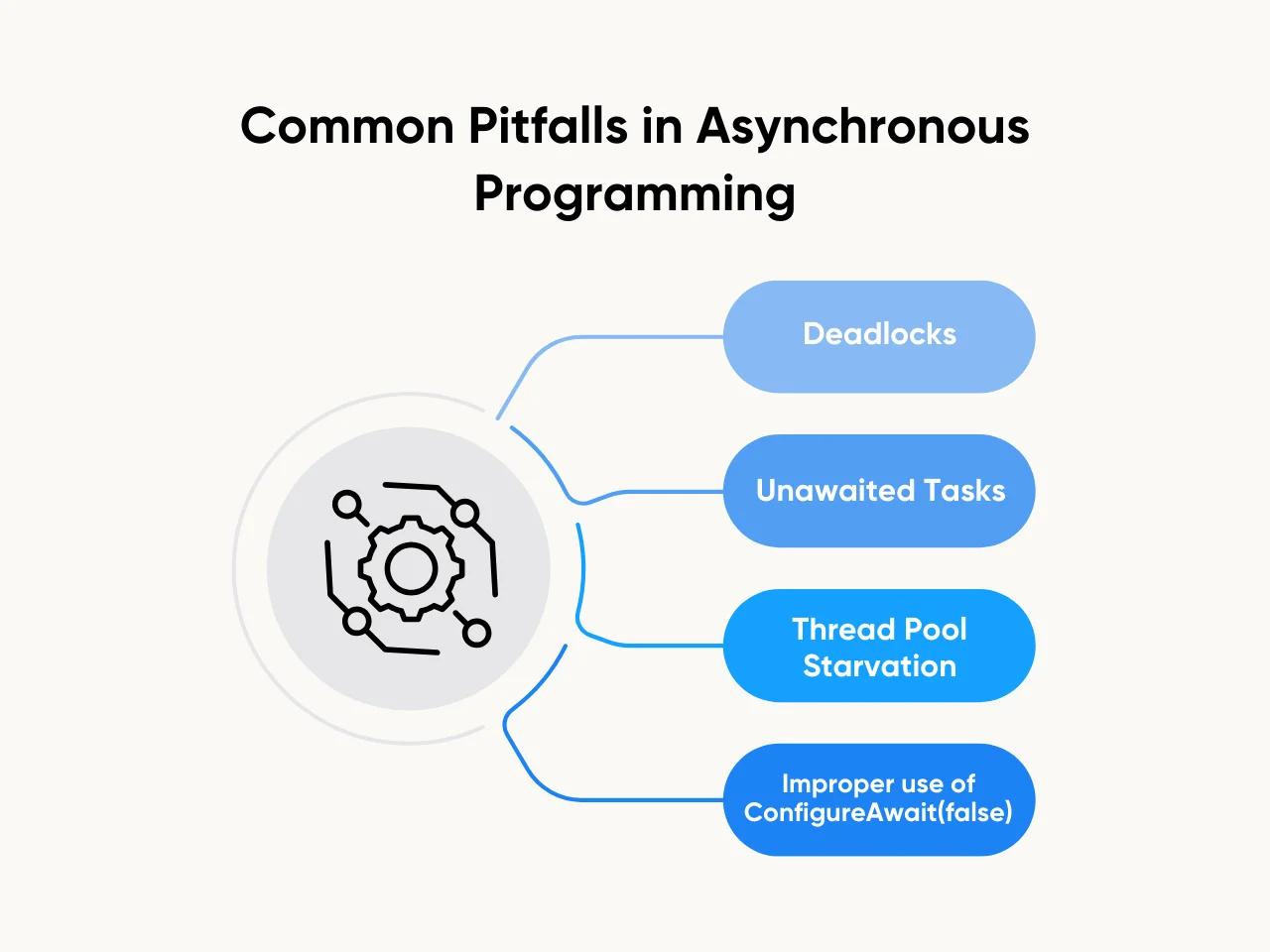
- Deadlocks: Occur when the main thread waits on a blocked async task. Avoid using .Result or .Wait(); prefer await to ensure non-blocking execution.
- Unawaited Tasks: Forgetting to await an async method can cause unintended behavior. Use tools to detect unawaited calls.
- Thread Pool Starvation: Overloading the thread pool leads to performance issues. Limit synchronous code inside async methods.
- Improper use of ConfigureAwait(false): Reduces context switching but may cause unexpected UI behavior in member functions of UI apps. Use it only for static objects or non-UI work.
When done right, asynchronous programming improves performance. Handling these pitfalls ensures optimized, reliable solutions for C# interview questions. Mastery of async programming and related topics like garbage collector behavior is vital for standing out in senior-level interviews.
Real-World Problem-Solving C# Interview Questions
Technical roles demand the ability to solve practical challenges through C# interview questions focusing on real-world problem-solving. From implementing the singleton design pattern to optimizing data structures and managing heap memory, these questions evaluate your approach to building efficient solutions while ensuring seamless software development processes.
1. How would you design a thread-safe singleton in C#?
In C# interview questions, designing a thread-safe singleton is a critical topic. A singleton ensures that only one instance of the class exists throughout an application’s lifecycle. Using Lazy<T> or double-checked locking provides robust solutions for thread safety. Lazy<T> initializes the class object only when needed, reducing unnecessary memory space usage. Double-checked locking uses synchronization to avoid creating multiple instances of a class in multi-threaded environments.
Singletons are appropriate in specific scenarios, including:
- Managing Global States: Singletons work well for logging frameworks or configurations, where a single static object ensures consistency across the application.
- Resource Management: A singleton ensures a single connection pool instance for tasks like database connections, reducing overhead and saving memory space.
- Caching Mechanisms: Using singletons for caching avoids creating a new object repeatedly, optimizing performance in high-frequency operations.
Potential drawbacks of singletons include:
- Tight Coupling: Dependency on a singleton can reduce modularity and make unit testing challenging.
- Global State Management Issues: A shared mutable state introduces risks in multi-threaded applications.
For senior-level C# interview questions, understanding thread-safe singleton patterns demonstrates expertise in balancing performance, scalability, and maintainability while addressing real-world problems.
2. What are the key principles of SOLID in object-oriented programming, and how do they apply in C#?
Understanding the SOLID principles is crucial for tackling real-world problem-solving C# interview questions. Each principle ensures a structured and maintainable codebase. In practice, adhering to SOLID principles enhances maintainability and scalability. For example:
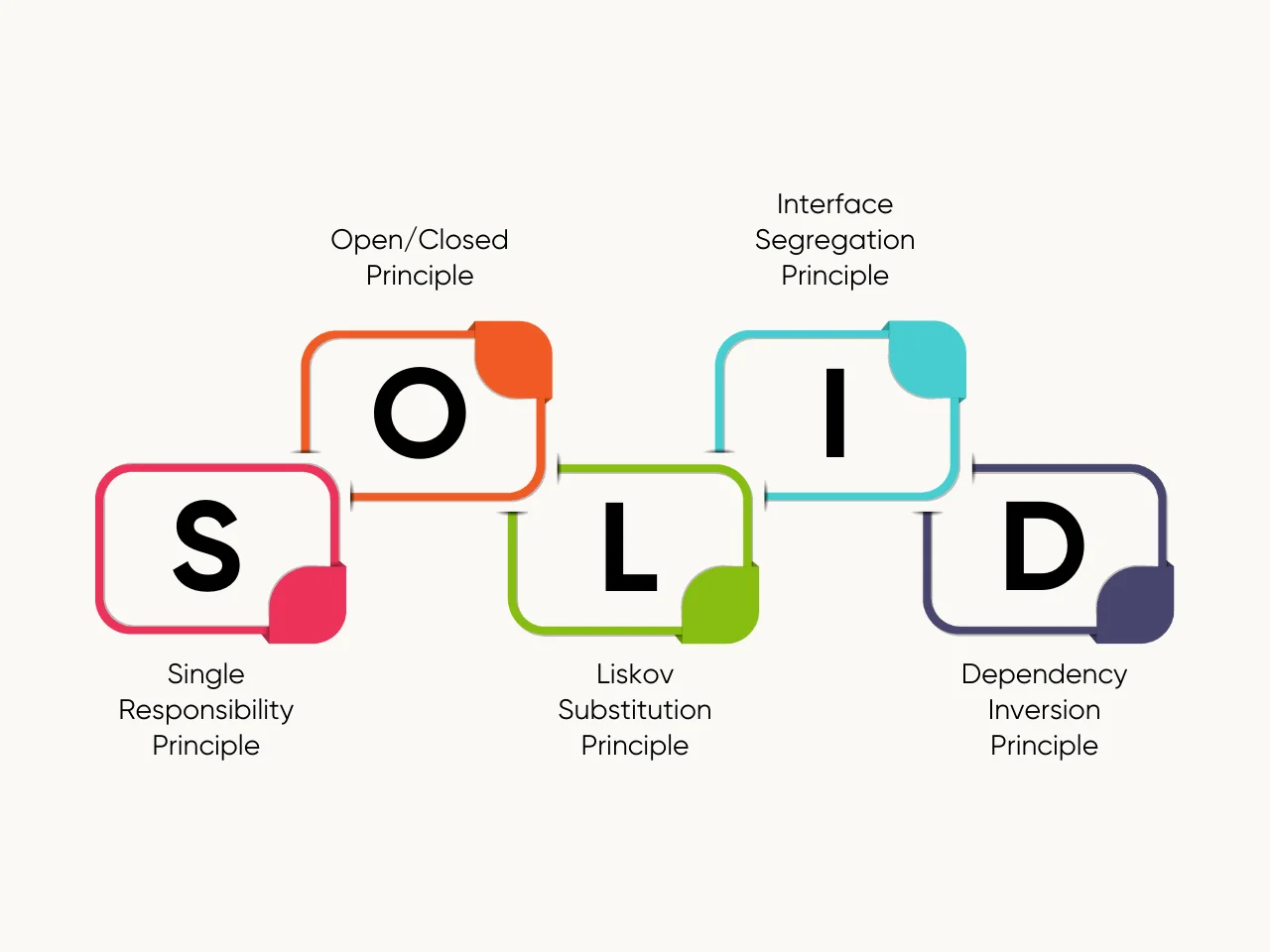
- Single Responsibility Principle: A logging system can use partial classes to separate file logging from error handling, avoiding unnecessary coupling.
- Open/Closed Principle: Extending a parent class for additional features preserves the core logic of the child class.
- Liskov Substitution Principle (LSP): Subclasses should be replaceable for their base classes without altering the program's correctness. For instance, substituting a derived class instead of its parent class should not cause unexpected behaviors.
- Interface Segregation Principle (ISP): Splitting interfaces into smaller, more focused units supports efficient use in different contexts.
- Dependency Inversion Principle (DIP): Relying on abstractions, such as an abstract class, rather than static objects, simplifies testing and dependency management.
Applying SOLID in C# interview questions demonstrates a candidate's ability to create robust, future-proof systems. These principles are especially relevant for managing real-world problem-solving C# interview questions, showcasing an understanding of flexible and reliable architecture.
3. How would you handle dependency injection in a C# application?
Dependency injection (DI) is a critical concept often discussed in real-world problem-solving C# interview questions. DI simplifies code modularity and enhances testability by decoupling object dependencies. Instead of hardcoding a dependency in a class object, DI injects it during runtime. This approach reduces tightly coupled code and promotes reusable components. For example, using Microsoft.Extensions.DependencyInjection allows seamless injection of services, improving maintainability.
Frameworks like Autofac streamline DI implementation for C# interview questions focused on architecture. DI ensures flexibility when replacing or testing member functions. Mastery of this concept demonstrates expertise in building scalable, maintainable applications during real-world problem-solving C# interview questions.
4. How do you manage performance in a large-scale C# application?
Managing performance in large-scale applications is a critical focus for senior developers in C# interview questions. Tools like .NET Performance Profiler and dotTrace help identify bottlenecks, enabling developers to optimize memory usage and improve execution speed. Using these profiling tools, developers can monitor instances of a class, analyze memory space utilization, and refine resource-heavy member functions.
To enhance performance, consider these techniques:

- Span<T> and Memory<T>: These tools optimize the stream of bytes and reduce heap allocation, improving the efficiency of file-handling operations.
- LINQ Query Optimization: Avoid deferred execution where unnecessary, and use foreach loops or projections that target specific value pairs in large datasets.
- Static Objects: To save memory and minimize repeated instantiations, especially for derived classes or reusable operations involving the string class.
Effective performance management involves a balance of efficient memory use, streamlined computations, and minimal overhead. Mastering these concepts demonstrates your readiness to tackle real-world C# interview questions. Interviewers often focus on tools, techniques, and your ability to handle advanced concepts like garbage collector optimizations and partial classes in complex systems.
5. How do you implement event-driven architecture in C#?
Event-driven architecture in C# relies on delegates, events, and the observer pattern. Delegates act as type-safe pointers to methods, while events allow communication between a class object and its listeners. The observer pattern connects subscribers to a publisher, ensuring updates when the state changes. For example, the foreach loop can iterate over subscribers to invoke methods dynamically, enhancing scalability.
Building a publisher-subscriber model involves defining an event using EventHandler or custom event arguments. This approach decouples logic, making systems modular and testable. Such implementation is commonly used in file handling, GUIs, or data science workflows.
Advanced .NET and Framework-Specific C# Interview Questions
Understanding the .NET framework's intricate workings is vital for excelling in C# interview questions. Senior developers must master concepts like the runtime environment, memory management, and leveraging managed code to ensure efficient program execution. Here are five key questions to help you showcase your expertise in runtime environments, type safety, and software development.
1. How does the .NET runtime handle assembly loading?
Assemblies load into the AppDomain, serving as the execution context for .NET applications. The runtime resolves assembly dependencies using metadata and binding information within the source code. It identifies assemblies through paths, names, and versions, converting the managed source code into machine code. Assemblies act as a single unit that houses types of classes, members of a class, and abstract methods.
To manage assemblies effectively, developers can use tools like:
- Assembly.Load(): Dynamically loads assemblies at run time, allowing the creation of types of classes, invoking extension methods, and ensuring null values are avoided.
- Global Assembly Cache (GAC): This cache stores shared assemblies in a centralized location to ensure efficient access across the operating system.
Mastering assembly loading prepares developers to handle advanced tasks like managing string objects, implementing constructor chaining, and dealing with null values. This showcases an understanding of different data types, read-only keywords, and automatic memory management, which are crucial for .NET development success.
2. What are ValueTask and Task, and when should you use them?
ValueTask and Task play vital roles in asynchronous programming. Task allocates memory for every operation, leading to higher memory space usage when creating multiple class instances. In contrast, ValueTask avoids allocation in certain cases, making it efficient for high-frequency tasks. Using ValueTask saves memory space when returning a result immediately, whereas Task is ideal for scenarios requiring multiple awaited operations.
ValueTask proves beneficial in specific scenarios, including:
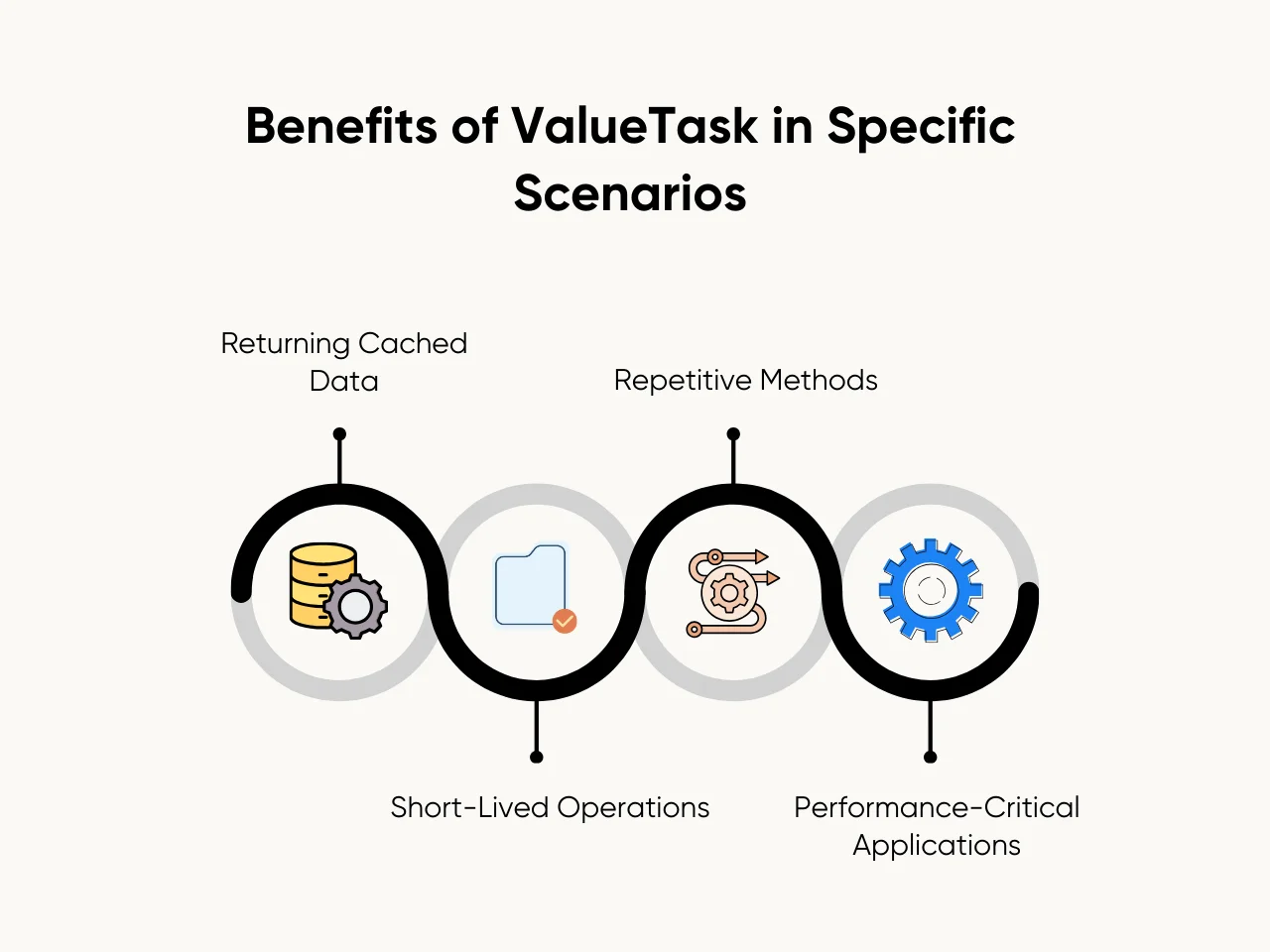
- Returning Cached Data: When results exist already, ValueTask avoids allocating a new object unnecessarily.
- Short-Lived Operations: Scenarios like quick file reads using file handling benefit from lower overhead.
- Repetitive Methods: Methods frequently invoked with minimal computation, such as formatting data using the string or a stringbuilder class.
- Performance-Critical Applications: Efficient for real-time systems like data science pipelines, where reducing allocation enhances throughput.
Understanding ValueTask and Task allows developers to optimize memory space and performance effectively. Selecting the right tool depends on the operation's lifecycle, balancing performance needs and readability. An interviewer may assess knowledge of these tasks alongside related concepts like early and late binding and how special methods or partial classes impact application architecture.
3. What is the role of reflection in C#, and what are its trade-offs?
Reflection in C# enables the inspection and manipulation of code during runtime. Developers use it to explore metadata, retrieve information about a class object, and dynamically interact with special methods or properties. For example, reflection allows examining an instance of the class, including its access modifiers, member functions, and new methods added during development. This flexibility aids in creating dynamic behaviors that adapt without recompilation.
Practical applications of reflection include:

- Dynamic Plugin Systems: Load and execute derived classes and plugins dynamically at runtime.
- Serialization Libraries: Convert an array of arrays or objects into a stream of bytes for data storage.
- Data Science Utilities: Manipulate value pairs or process jagged arrays effectively.
- Testing Frameworks: Invoke single methods and automate testing tasks with partial classes.
- File Handling: Use reflection to map properties to StringBuilder class elements for better data output.
While reflection provides versatility, it has trade-offs. The garbage collector may face increased strain due to static objects and new objects created dynamically, impacting memory space. Reflection also introduces performance overhead, delays compile-time execution, and reduces type safety, as late binding may cause runtime errors compared to early binding.
4. What is dependency property in WPF, and how does it differ from CLR properties?
Dependency properties in WPF enable advanced features like data binding, animations, and dynamic styling, making them essential for creating flexible and responsive UIs. Unlike CLR properties, dependency properties rely on the WPF property system, which tracks changes and provides enhanced functionality such as default values, value inheritance, and change notifications.
To create a custom dependency property, developers define it as a static field in a class and register it using the DependencyProperty.Register method. For instance, a custom FontSize property in a class object can dynamically respond to style changes or updates, unlike standard CLR properties.
5. How do you ensure compatibility when building libraries targeting multiple .NET versions?
Ensuring library compatibility across multiple .NET versions involves strategies like multi-targeting. Using .NET Standard allows developers to write code compatible with various frameworks, while .NET 5/6 provides enhanced features for unified application development. Multi-targeting ensures a library functions seamlessly across platforms, reducing duplication.
Conditional compilation (#if) offers a way to include version-specific code. Defining preprocessor directives, developers can isolate features or APIs unique to a particular .NET version. This approach ensures that applications leveraging the library remain functional and optimized regardless of the framework version.
Key Takeaway
C# interview questions for senior roles test technical expertise and problem-solving ability. Employers seek developers who understand advanced concepts like asynchronous programming, garbage collection, and SOLID principles. Mastering these topics ensures confidence and readiness for challenging interviews. Stay updated on new trends to stay ahead of the competition.
Hiring tech practices emphasize expertise and adaptability. Trends in hiring app developers show that businesses prioritize candidates with strong coding skills and practical experience. Employers also value the ability to integrate new technologies seamlessly into existing systems. Preparing for C# interview questions focusing on practical applications gives you a competitive edge.
Are you looking to level up your skills with C# interview questions? At Aloa, we guide you through the software development process, connecting you with vetted experts and advanced tools to achieve your goals. Explore our transparent project management framework to ensure success in every step of your development journey.