React is a powerful JavaScript library that dominates web development. Its popularity stems from features like React hooks, unidirectional data flow, and the virtual DOM, which enable efficient handling of data changes and state management in web applications. As a developer, mastering React interview questions ensures a strong grasp of topics like reusable components, JSX elements, and React features like the component lifecycle and context API.
We at Aloa understand the needs of modern developers. Aloa is a software outsourcing agency that excels in creating web applications using advanced tools like React. Through a robust project management framework, we connect clients with the best software engineers who utilize React native, CSS modules, and JavaScript frameworks. With our guidance, your React application will leverage component logic, pure functions, and scalable state management.
Building on our expertise, this guide tackles React interview questions covering beginner to advanced topics. You’ll explore the representation of the actual DOM, React fragments, prop drilling, and optimizing the state of a component. By the end, you’ll understand how to use higher-order components, implement error boundaries, and optimize a React app with lazy loading and redux store techniques.
Let's get started!
Intermediate-Level React Interview Questions
React's flexibility and powerful features make it essential for web development. At the intermediate level, interviews often explore React hooks, state management, and optimizing the component tree. Here are the six intermediate-level React interview questions you should prepare for, with insights into key React features and best practices.
1. What are React hooks, and why are they important?
React hooks transformed the way developers work with React components, enabling the shift from class components to function components. Introduced to simplify the management of state changes and side effects, hooks eliminated the need for lifecycle methods in many scenarios. This improvement allowed developers to write cleaner, reusable javascript code while building a better user interface.
Key hooks include:
- useState: This manages the local state of a React component. It tracks changes within the javascript background, such as updating the value of a child component or toggling a controlled component.
- useEffect: This handles side effects like fetching data or interacting with the real DOM. It replaces lifecycle methods componentDidMount for functional components.
Example Follow-Up Question: Explain the dependency array in the useEffect hook. The array ensures that the effect runs only when its specified dependencies, like a value of the parent component, change. Without this array, the effect would run after every state change or render.
Using hooks like useState and useEffect enhances React's features, allowing developers to create seamless Javascript applications with better performance. Understanding these concepts helps when answering React interview questions about components, render methods, and even fallback UI.
2. What is the significance of the key prop in React lists?
The key prop in React plays a crucial role in maintaining an application's efficiency. When rendering lists, React uses the key prop to track which items have changed, been added, or removed during updates. This tracking ensures smooth and accurate rendering of HTML elements, optimizing performance and avoiding unnecessary re-renders.
Unique keys are essential to avoid rendering issues. Here are some best practices for creating keys in React lists:
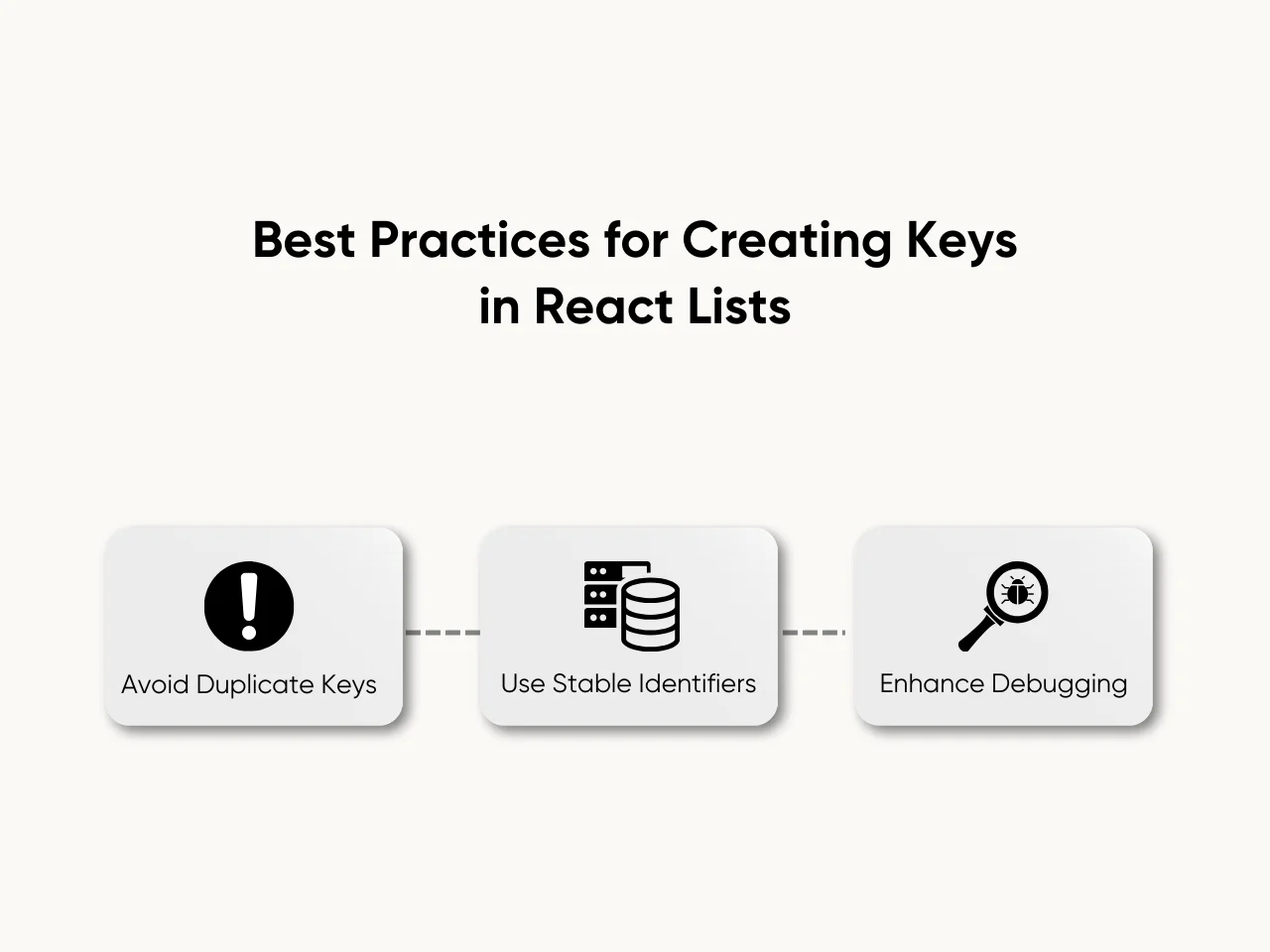
- Avoid Duplicate Keys: Duplicate keys in a list can cause React to misidentify items, leading to rendering errors.
- Use Stable Identifiers: Use unique IDs or database keys instead of indices to ensure stability in dynamic lists.
- Enhance Debugging: Unique keys, such as React logs warnings for missing or duplicate keys, make debugging easier.
When asked about the key prop in React interview questions, interviewers expect detailed and practical answers. Discuss how the key prop impacts features of React, such as reconciliation and performance. Reference its role in advanced concepts like stateless components, render props, and higher-order components. Additionally, it should be mentioned in a React application that uses a react-router, uncontrolled components, and single-store state management.
Prepare to address follow-ups, like writing a dynamic JavaScript function to assign keys or demonstrating event handlers that modify lists. Highlight its compatibility with regular JavaScript, arrow functions, and JavaScript XML (JSX). Referring to Jordan Walke’s vision for React, emphasize how the key prop enhances modular development and reusable new components.
4. How does conditional rendering work in React?
Conditional rendering allows React to display elements dynamically based on specific conditions. This feature enhances interactivity in a React application, helping developers customize what users see. React evaluates conditions using regular JavaScript logic and updates the UI accordingly. This flexibility enables seamless integration with React features like state and props to manage user interfaces efficiently.
Conditional rendering techniques include the following:

- If-Else Statements: Use this structure to render one of multiple HTML elements within a component.
- Ternary Operators: Inline conditions simplify logic, offering a concise way to toggle between elements. Example: {isLoggedIn ? "Welcome" : "Guest"}.
- Logical Operators (&&): Render elements only when a condition is true, reducing code clutter. Example: {isAdmin && <AdminPanel />}.
An answer for these React interview questions should clearly explain when and how to use each technique. Candidates should explain their reasoning, provide concise code snippets, and demonstrate practical applications. Strong answers will also highlight the benefits of each method for maintaining clean, efficient code in React applications.
5. What are controlled and uncontrolled components in React forms?
Controlled and uncontrolled components form the backbone of managing forms in React. Understanding their differences helps in choosing the right approach for specific requirements. React interview questions often focus on explaining these concepts clearly with examples to demonstrate practical knowledge. Here’s the breakdown:
- Controlled components: React state manages these elements. Real-time control enables seamless validation and updates. For instance, an input field bound to a state variable updates instantly with every user change.
- Uncontrolled components: The DOM handles these elements directly. Developers access their values through refs for minimal React state interference, making them ideal for simple or third-party integrations.
Use cases differ based on complexity. Controlled components work best for forms needing live validation or dynamic behavior. Uncontrolled components are ideal for simple forms where managing the state isn’t critical.
The developer should highlight that controlled components provide superior control and validation, while uncontrolled components simplify implementation for less critical tasks. An ideal response might include examples showing controlled input for forms requiring live error checks and uncontrolled input for non-critical data like uploading a file.
6. Explain React’s Context API and its use cases.
The Context API simplifies state management in React applications. It eliminates the need for "prop drilling," allowing components to share global states directly without passing props through multiple layers. This feature improves code readability and maintains cleaner component structures, which often come up in React interview questions.
Here are examples of Context API use cases:
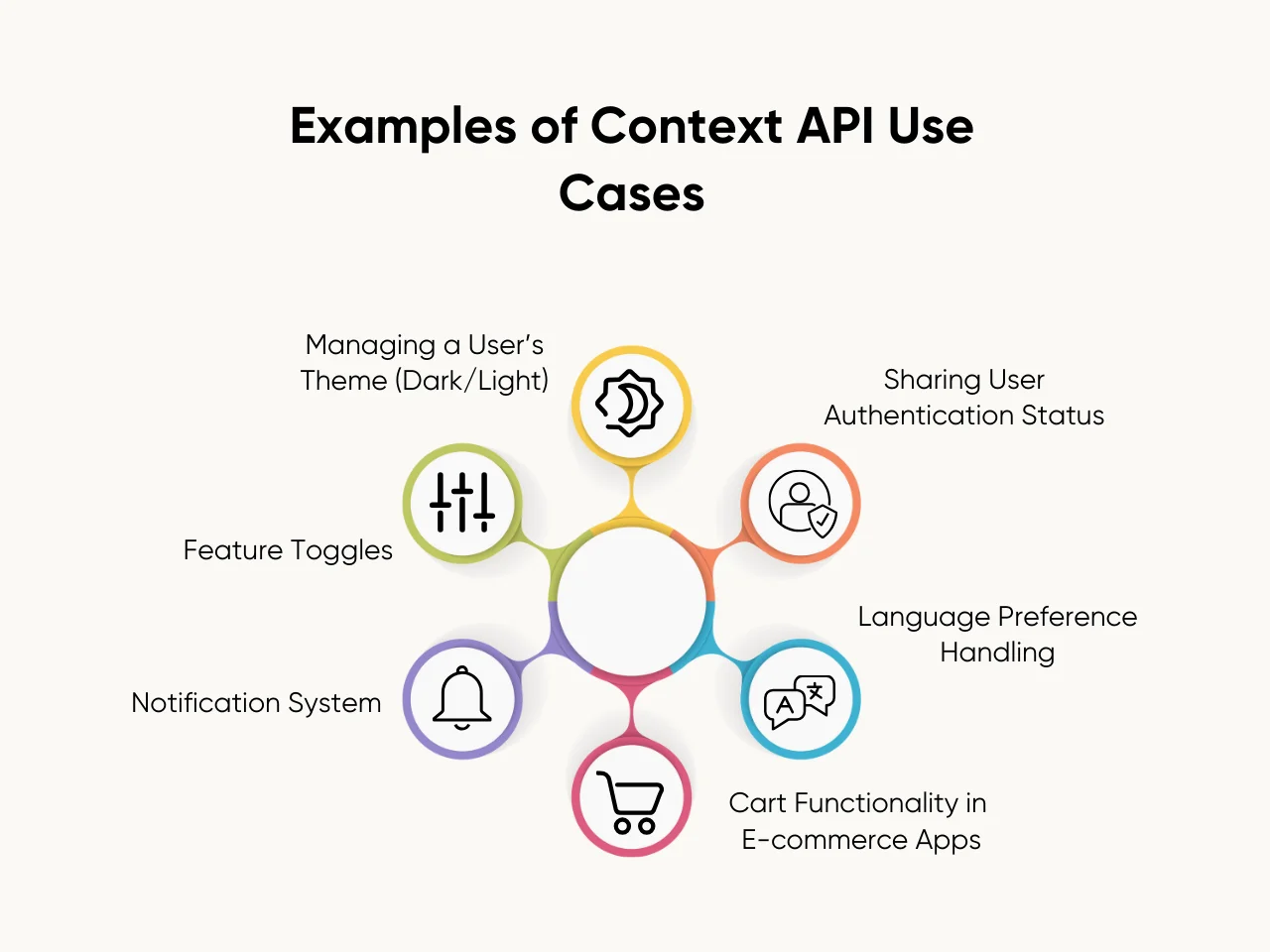
- Managing a User’s Theme (Dark/Light): The Context API can store and switch themes dynamically, ensuring consistent styling across the app.
- Sharing User Authentication Status: It enables seamless access to authentication details, like whether a user is logged in or their role (admin, user), across components.
- Language Preference Handling: Context helps manage multilingual support by storing and updating the app's language setting.
- Cart Functionality in E-commerce Apps: Components can access and update the shopping cart state without prop dependencies.
- Notification System: A global state for managing toast or in-app notifications can use Context to ensure instant page updates.
- Feature Toggles: Context allows tracking and toggling feature flags dynamically in larger applications.
Candidates should explain how the Context API provides a centralized, efficient state-sharing mechanism with practical examples. They should mention its advantages in reducing prop complexity while maintaining flexibility in global state management.
7. How does React’s reconciliation process work?
React's reconciliation process ensures efficient updates to the user interface. This optimization revolves around the virtual DOM, a lightweight representation of the real DOM. React compares the virtual DOM with its previous version to identify necessary changes, avoiding fully re-rendering the real DOM.
Here are the key steps in reconciliation:
- Diffing Algorithm for Detecting Changes: React compares the current virtual DOM with the previous version. It identifies changes, additions, or deletions in components and their properties.
- Efficient Updates to the DOM via Batch Rendering: React batches multiple updates into a single operation, reducing the number of interactions with the real DOM. This minimizes performance bottlenecks and ensures a smoother user experience.
The developer should explain how React leverages the virtual DOM to enhance performance. An ideal response includes examples, such as updating only modified components (e.g., a list with a new item) while leaving unaffected components unchanged.
Advanced-Level React Interview Questions
React's advanced topics dive deep into its capabilities for building scalable, high-performing web applications. For senior roles, mastering concepts like server-side rendering, higher-order components, and handling complex application states. Here are the advanced-level React interview questions focusing on optimization, architecture, and advanced React features.
1. What are higher-order components (HOCs) in React?
Higher-order components (HOCs) are functions in React that take an existing component as an argument and return a new component with enhanced functionality. This design pattern allows for code reuse and is especially useful for adding common logic to multiple components without duplicating code.
Here are common use cases for HOCs:
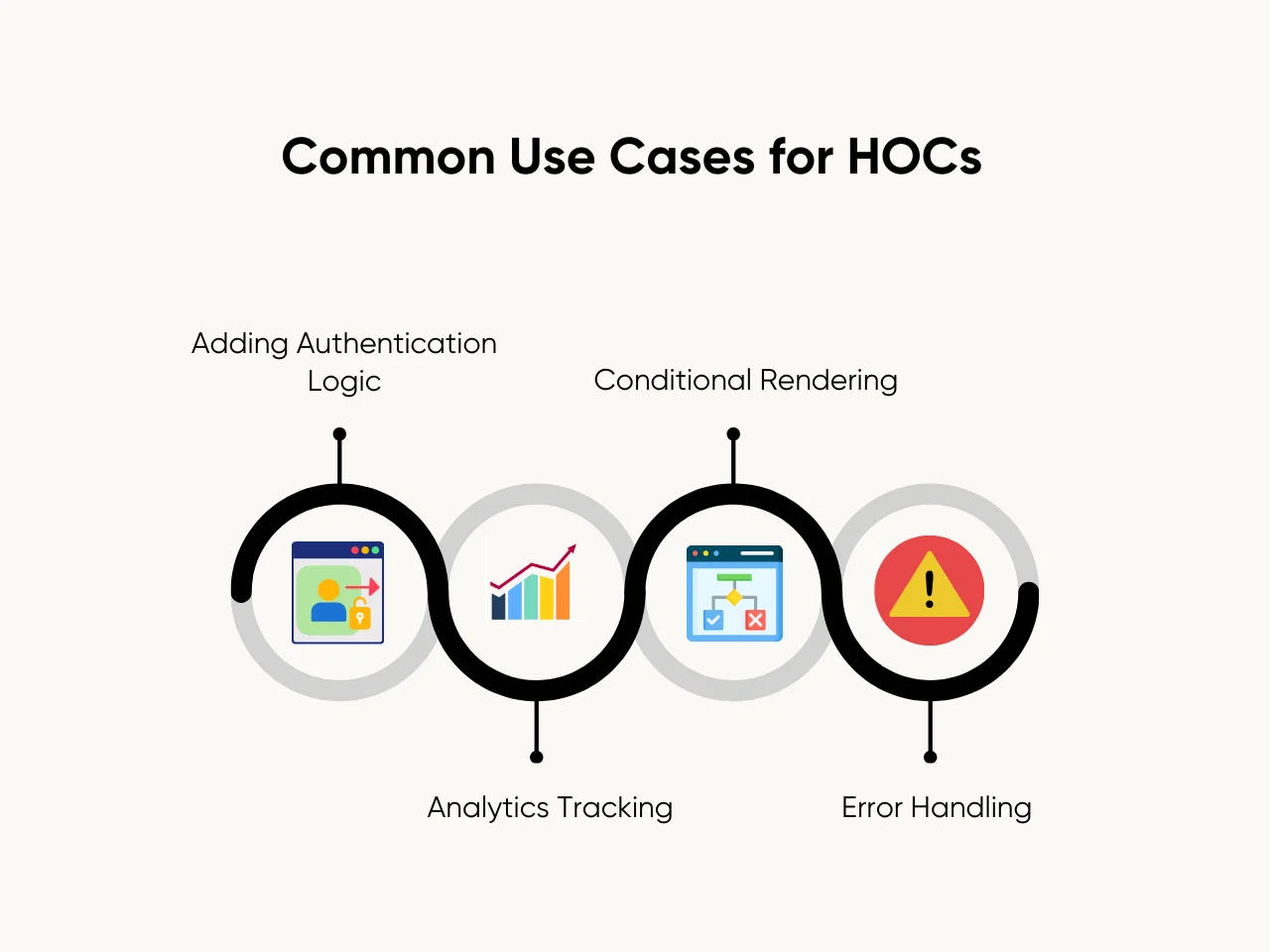
- Adding Authentication Logic: Protect routes or components by wrapping them with a function that checks user permissions.
- Analytics Tracking: Enhance components to log user interactions for tracking purposes.
- Conditional Rendering: Dynamically decide whether to show or hide components based on specific conditions.
- Error Handling: Wrap components to catch and display errors in a user-friendly way.
A response should include an example of a simple HOC. The candidate should describe how the HOC wraps a button component to log a message whenever the button is clicked. This example clearly illustrates the role of HOCs in enhancing component behavior without modifying the original component. Look for precise explanations and an understanding of how HOCs contribute to code modularity and maintainability.
2. How can you optimize the performance of a React application?
Optimizing the performance of a React application ensures smooth user experiences and efficient resource utilization. Developers employ several techniques to achieve this. These are the performance optimization techniques:
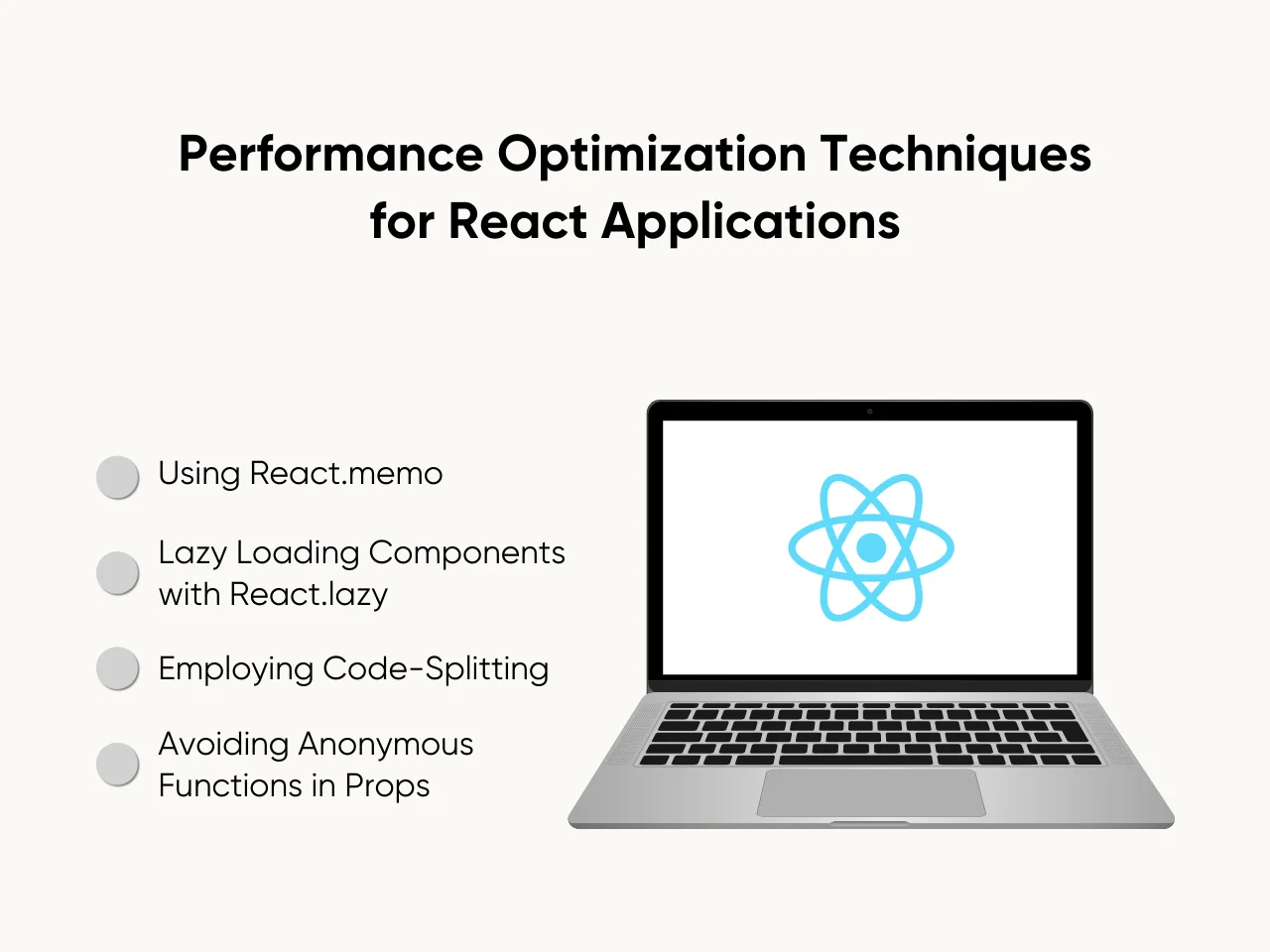
- Using React.memo: React.memo prevents unnecessary re-renders by memoizing components. It ensures components re-render only when their props change, enhancing efficiency.
- Lazy Loading Components with React.lazy: Lazy loading defers component loading until needed, reducing the initial bundle size and improving load times.
- Employing Code-Splitting: Code-splitting with tools like Webpack separates the application’s code into smaller chunks. It ensures users download only the required parts, speeding up initial page loads.
- Avoiding Anonymous Functions in Props: Passing anonymous functions as props create new references on every render. Using named functions or useCallback helps maintain consistent references and minimizes re-renders.
An ideal response includes explaining these techniques with practical examples, such as implementing React.memo or demonstrating code-splitting. Candidates should showcase how these strategies reduce load times, optimize rendering, and enhance overall application performance.
3. What are custom hooks in React, and how are they created?
Custom hooks in React encapsulate reusable logic, allowing developers to share functionality across components without duplicating code. Custom hooks often use existing React hooks, such as useState or useEffect to handle state management or side effects. For example, a custom hook for API data fetching can manage the loading state, fetch data, and handle errors, providing a clean and consistent way to perform this operation in multiple components.
The benefits of custom hooks are significant. They reduce repetitive code, making applications more straightforward to read and maintain. Custom hooks adhere to the DRY (Don't Repeat Yourself) principle, ensuring logic updates are centralized and streamlined. Additionally, custom hooks improve code organization by separating logic from the UI layer, making components more focused and testable. This approach simplifies scaling applications as shared functionality grows.
4. What is server-side rendering (SSR), and how does it differ from client-side rendering?
Server-side rendering (SSR) involves pre-rendering HTML on the server and delivering fully-formed pages to the browser. This approach improves page load speed and enhances SEO because search engines can easily crawl pre-rendered content. SSR benefits applications that rely on dynamic content or need quick initial load times to improve user engagement.
In contrast, client-side rendering (CSR) generates content dynamically in the browser after loading JavaScript. While CSR can make applications feel interactive and modern, it often results in slower initial load times, especially for users with slower devices or connections. The browser must first download and execute JavaScript before displaying any content.
Frameworks like Next.js simplify implementing SSR in React applications. They combine the benefits of SSR for initial page loads with CSR’s dynamic capabilities, enabling developers to optimize performance and deliver a better user experience.
5. How do you handle complex state management in a React application?
Managing a complex state in a React application requires selecting the right tools and strategies based on the application’s requirements. While React provides built-in options like the Context API and useReducer hook for state management, external libraries can simplify handling more advanced or large-scale scenarios.
Here are the popular libraries for state management:

- Redux: Best suited for large-scale applications with deeply nested components and complex interactions. Redux centralizes the state, making it easier to debug and maintain.
- Zustand: A lightweight alternative to Redux. It offers simplicity and flexibility and is suitable for medium-sized applications needing a straightforward state management solution.
- React Query: Focused on server-state management. It excels at handling data fetching, caching, and synchronization with APIs.
For simpler apps, Context API or useReducer is sufficient. In applications requiring advanced state management or server-state handling, libraries like Redux or React Query become essential. Each tool has its strengths, and the choice depends on the complexity and requirements of the application.
Behavioral and Problem-Solving React Interview Questions
Behavioral and problem-solving questions assess your ability to apply React features in real-world scenarios. Expect to discuss component lifecycle, debugging techniques, and challenges like managing data changes or avoiding prop drilling. Here are the behavioral and problem-solving React interview questions to showcase your expertise in solving React application challenges.
1. Can you describe a challenging React project you worked on and how you resolved issues?
A well-crafted answer to this question highlights problem-solving skills and expertise in handling React challenges. Candidates should choose a project where they faced a specific issue and successfully resolved it using strategic thinking. Examples include optimizing performance, managing state complexity, or integrating third-party libraries effectively.
When structuring responses, use the STAR method:
- Situation: Begin with a clear explanation of the project context and challenges.
- Task: Define the specific goals and responsibilities involved.
- Action: Detail the steps to overcome the challenges, focusing on React techniques or tools.
- Result: Highlight the positive outcomes, including metrics or qualitative improvements.
An example involves migrating a legacy app to React while ensuring backward compatibility. This process may involve replacing outdated components, optimizing the app’s structure, and thoroughly testing to maintain functionality across older systems. Managing such transitions demonstrates knowledge of React’s flexibility and ability to deliver efficient solutions.
2. How do you debug a React application?
Debugging a React application requires a methodical approach to identify and resolve issues efficiently. With the right tools and techniques, developers can ensure the application runs smoothly and delivers a seamless user experience.
Here are the techniques for debugging React applications:
- React Developer Tools: Use this browser extension to inspect React components and their states. It allows you to traverse the component tree, monitor props and state values, and identify discrepancies in data flow.
- Console Logging and Breakpoints: Insert console.log() statements to track variables and events during runtime. Use Chrome DevTools to set breakpoints in the code, enabling step-by-step execution to pinpoint errors.
- Profiler Tab: Leverage the Profiler tab in React Developer Tools to identify performance bottlenecks. This tool provides insights into component rendering times and helps optimize rendering cycles for better performance.
Debugging React applications involves understanding tools like React Developer Tools, Chrome DevTools, and the Profiler tab. Applying these techniques ensures faster issue resolution and improves the overall quality of the application.
3. How do you handle API errors in React?
Handling API errors in React involves implementing strategies that ensure the application remains user-friendly and functional even when issues occur. Proper error management improves user experience and application reliability.
Follow these strategies for managing API errors in React:
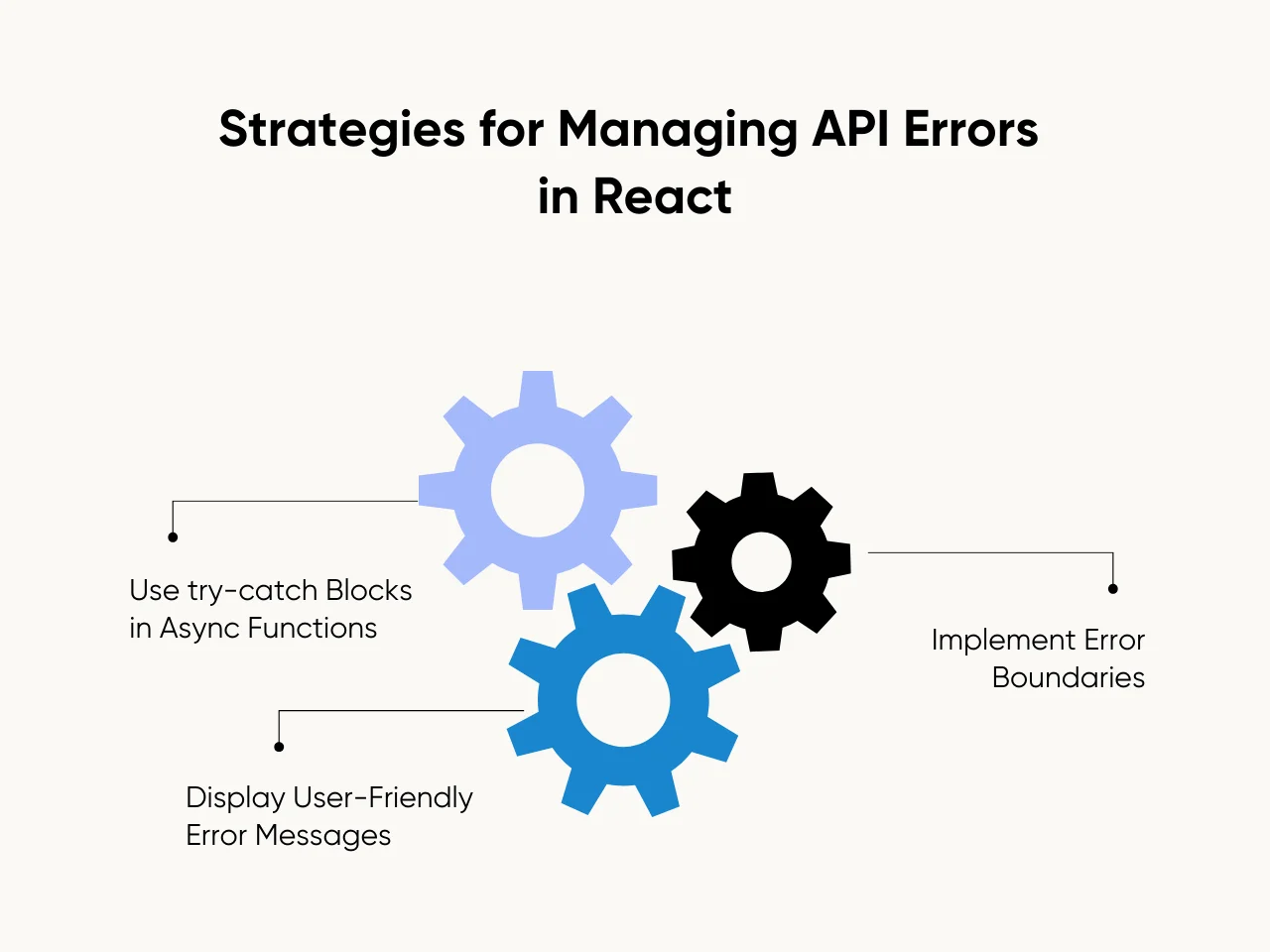
- Use try-catch Blocks in Async Functions: Wrap asynchronous API calls in try-catch blocks to catch and handle errors effectively. This approach allows you to identify the error type and decide how to respond, such as retrying the request or logging the issue.
- Implement Error Boundaries: For rendering errors, use React's error boundaries. These components catch errors in the component tree and display fallback UI without crashing the entire application. While error boundaries cannot handle async errors, they ensure a smooth user experience during rendering issues.
- Display User-Friendly Error Messages: Replace technical error details with clear, understandable messages for users. For example, a message like "Something went wrong. Please try again later" should be shown to maintain professionalism and reassure users.
Effectively managing API errors in React involves a combination of defensive programming and user-focused practices. Leveraging try-catch blocks, error boundaries, and intuitive messaging ensures errors are handled gracefully. This approach prevents disruptions and builds trust with users through proactive communication and a stable interface.
4. How would you implement authentication in a React app?
Implementing authentication in a React app often involves using JWTs (JSON Web Tokens) for secure user authentication and authorization. After a user logs in, the server generates a token that the app stores in cookies or local storage. The token is included in API requests to verify the user's identity and grant access to protected resources. This ensures seamless session management within the app.
Securing APIs and managing token expiration are critical steps in the process. Always validate tokens on the server side and refresh them as needed to prevent unauthorized access. Employ HTTPS and secure storage practices to safeguard sensitive data and maintain user trust.
5. How do you optimize React for SEO purposes?
Optimizing React for SEO involves addressing challenges caused by JavaScript-heavy applications that search engines might need help to index effectively. Implementing server-side rendering (SSR), static site generation (SSG), and proper meta-tag management enhances discoverability and improves search rankings.
Here are techniques for optimizing React for SEO:

- Server-Side Rendering (SSR): Generate HTML on the server to ensure search engines can index fully rendered pages, improving performance and SEO. Tools like Next.js simplify SSR in React apps.
- Static Site Generation (SSG): This method pre-renders pages at build time to enhance loading speed and provide easily crawlable content. It is ideal for static or rarely updated sites.
- Meta Tag Management with React Helmet: Dynamically set meta tags like titles, descriptions, and canonical URLs to ensure each page is optimized for search engines.
Combining SSR, SSG, and efficient meta tag management ensures a React application is optimized for SEO. These techniques improve crawlability, enhance user experience, and help achieve higher search engine rankings, making them essential for any React-based project focused on visibility and performance.
Key Takeaway
React interview questions are essential for preparing developers to showcase their skills and knowledge. These questions test core concepts like React hooks, state management, and component lifecycles. Mastering these topics ensures candidates are ready to tackle challenges and demonstrate their expertise during interviews. Understanding these interview questions also helps developers build confidence, which is key in high-pressure scenarios.
Assessing skills plays a critical role in identifying the right talent for companies. These questions highlight a candidate’s ability to solve problems, write clean code, and work effectively with React's tools and libraries. Employers should focus on technical skills and the candidate’s approach to problem-solving when assessing responses to React interview questions.
Ready to take your team’s hiring process to the next level? At Aloa, we help businesses hire the best React developers through a streamlined process and expert guidance. Let us assist you in building a talented team equipped to meet your development needs. Contact us to learn more!